How to Skip Iterations in a Python Loop
-
Use the
try-except
Statement Withcontinue
to Skip Iterations in a Python Loop -
Use the
if-else
Statement Withcontinue
to Skip Iterations in a Python Loop
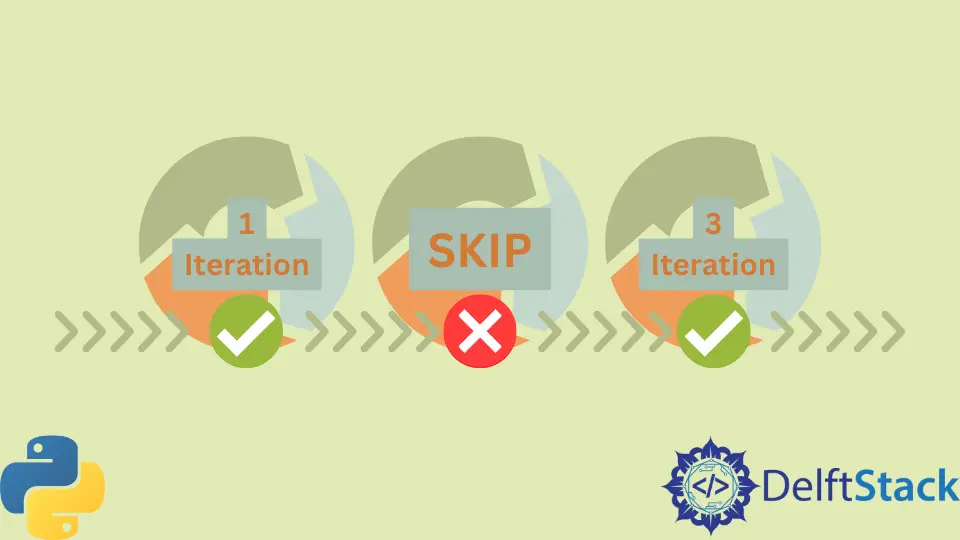
This article explains different ways to skip the specific iterations of a loop in Python.
Sometimes, we have to deal with the requirements of performing some tasks repeatedly while skipping a few of them in between. For example, when you are running a loop and want to skip the part of that iteration that can throw an exception.
Use the try-except
Statement With continue
to Skip Iterations in a Python Loop
In Python, exceptions can be easily handled through a try-except
statement. If you think that you may come across some exceptions during loop iterations, due to which the execution of the loop may stop, then you can use this statement.
List_A = [25, 30, 100, 600]
List_B = [5, 10, 0, 30]
Result = []
for i, dividend in enumerate(List_A):
try:
# perform the task
Result.append(dividend / List_B[i])
except:
# handle the exceptions
continue
print(Result)
In the above code, we have two lists, and we want to divide List_A
by List_B
element by element.
In Python, when you divide a number by zero, the ZeroDivisionError
occurs. Since List_B
contains zero as a divisor, division by it will generate this error during loop execution.
So to avoid this error, we use the except
block. The exception will be raised when the error occurs, and the except
block will be executed.
The continue
statement ignores any subsequent statements in the current loop iteration and returns to the top of the loop. This is how you can skip the loop iterations.
The above code generates the following output:
[5.0, 3.0, 20.0]
Use the if-else
Statement With continue
to Skip Iterations in a Python Loop
We can do the same task with an if-else
statement and continue
.
List_A = [25, 30, 100, 600]
List_B = [5, 10, 0, 30]
Result = []
for i, dividend in enumerate(List_A):
if List_B[i] != 0:
Result.append(dividend / List_B[i])
else:
continue
print(Result)
This is a straightforward code. The difference between this solution and the try-except
solution is that the former implementation already knows the condition for which the loop execution may get stopped.
Therefore, this condition can be explicitly coded to skip that iteration.
Output:
[5.0, 3.0, 20.0]
As a result of the above implementations, you can skip loop iteration on which error/exception may occur without the loop being halted.