How to Determine the Size of an Object in Python
-
the
sys
Module in Python -
Use the
getsizeof()
Function in thesys
Module to Get the Object Size in Python
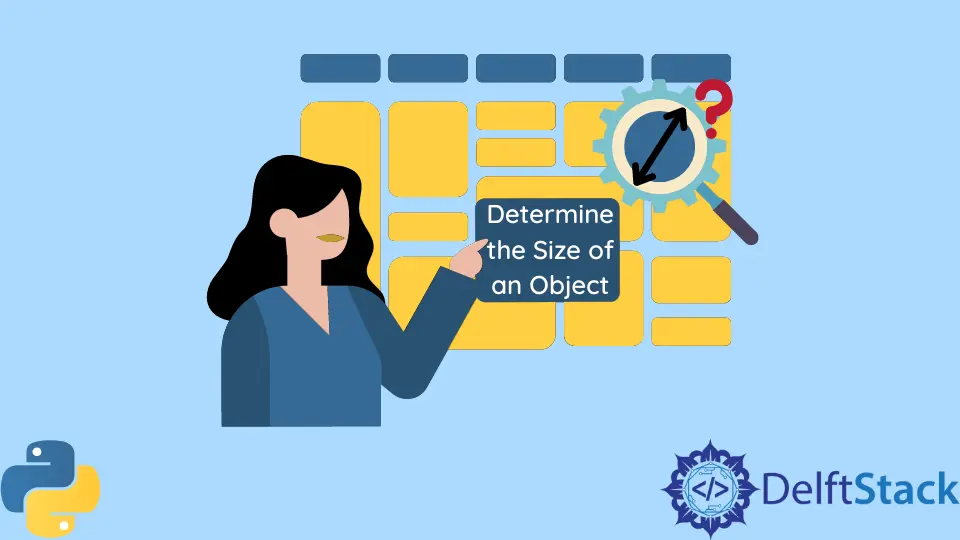
There are different built-in data types in programming like numeric, text, sequence, and boolean. In Python, all these data types are considered objects. Every object needs some space in the memory to store itself. So, these objects store themselves in the memory and occupy space in the form of bytes
. Every object has a different storage size, and this tutorial demonstrates how to find the size of an object in Python.
the sys
Module in Python
The sys
module of Python helps a user perform various operations and manipulations on various parts of the Python runtime environment by providing multiple functions and variables. One can easily interact with the interpreter through different variables and functions. While using the sys
module, you can easily access system-specific functions and parameters.
The sys
module is also used in determining the size of an object in Python.
Use the getsizeof()
Function in the sys
Module to Get the Object Size in Python
The getsizeof()
function provided by the sys
module is the most commonly used function to get the size of a particular object in Python. This function stores an object as its function argument, calls that object’s sizeof()
function, and finally returns the output.
import sys
s = sys.getsizeof("size of this string object")
print(s)
s = sys.getsizeof(1010101)
print(s)
s = sys.getsizeof({1: "size", 2: "of", 3: "this", 4: "dictionary"})
print(s)
Output:
63
24
280
Note that the sizes of the given objects returned are in bytes.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn