How to Get the String Length and Size in Python
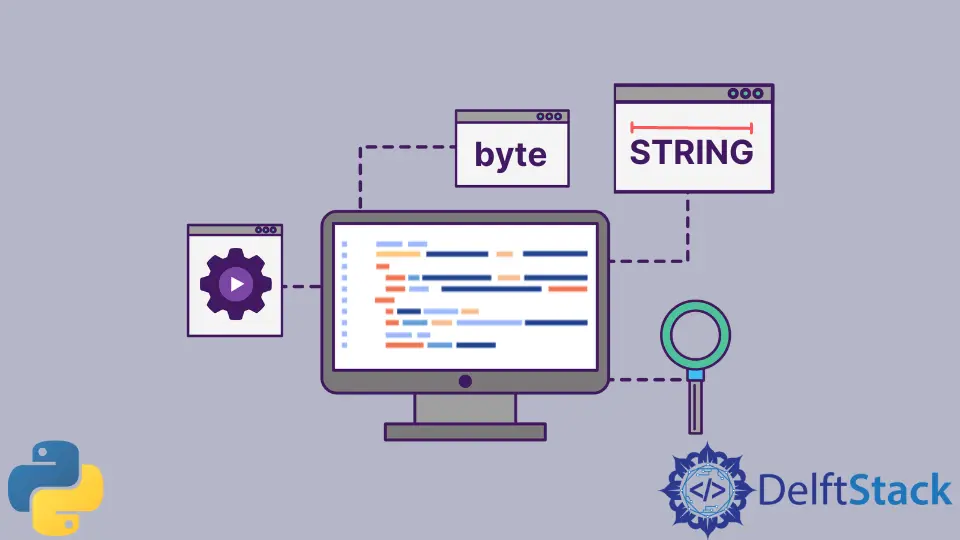
Strings are a prevalent data type and are used in almost every programming language. It is a collection of characters and can be considered as an array of characters.
In Python, anything enclosed between quotation marks, single or double, can be considered as a string.
This tutorial will introduce how to get the length and size of a string in Python.
Get the String Length in Python
We can create our little function to get the length of a string in Python. The function will iterate through every character in the string using a for
loop and increment a counter variable on every iteration until the string’s end is encountered. The following code implements this:
x = "Sample String"
length = 0
for i in x:
length += 1
print(length)
Output:
13
We can also use the len()
function to find the length of a string. For example:
x = "Sample String"
print(len(x))
Output:
13
Note that the len()
function may provide unwanted results at times; for example, it does not count \
character in escape sequences, as shown below.
print(len("\foo"))
Output:
3
Get the String Size in Python
The size of the object is the number of bytes in the memory allocated for this object. The sys
module has a function called getsizeof()
that returns an object’s memory size in bytes. See the following example code.
import sys
x = "Sample String"
print(sys.getsizeof(x))
Output:
62
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn