Shebang in Python
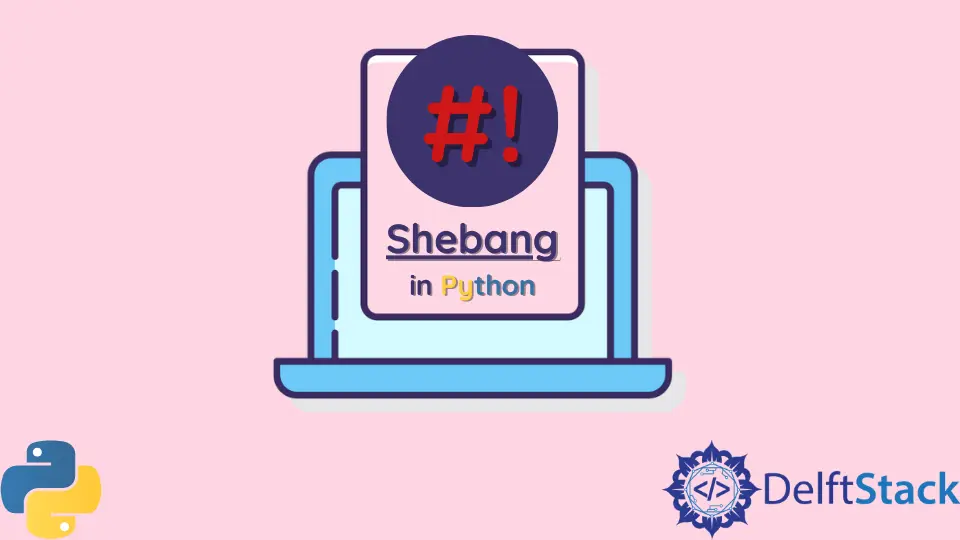
In this article, we’re going to talk about what shebang
means in Python and how you can use it in a command. We’ve included an example program to help you understand the concept of Python shebang
better.
shebang
Definition
The shebang
character sequence is a special character sequence in a script file denoted by #!
. It helps in specifying the type of program that should be called to run the entire script file. The shebang
character sequence is always used in the first line of any file.
The statement that mentions the program’s path is made by using the shebang
character first and then the path of the interpreter program.
shebang
in Python
For all the scripts that are to be executed in Python3, use the following command in the command line:
#!/usr/bin/env python3
Replace python3
with python2
if the script is only compatible with Python 2.7 version.
If the script is compatible with both Python 2 and Python 3, the following command can also be used:
#!/usr/bin/env python
This code is also understandable on Windows Python Launcher.
The program above is preferred when writing a shebang
command in a virtual environment like pyenv
. Previously known as Pythonbrew
, pyenv
is a simple Python version management tool that helps manage the Python version, install the latest Python versions, and create a virtual Python environment.
In most cases, a Python interpreter is installed at /usr/bin/python
or /bin/python
. In these two cases, the shebang
statement will fail if the following command is used:
#!/usr/local/bin/python
In conclusion, the shebang
command is just a way of denoting that the following script is executable. Therefore, the result of any python script will never be affected if the shebang
command is executed, whether it is mentioned or not.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn