How to Convert Set to String in Python
-
Use the
map()
andjoin()
Functions to Convert a Set to a String in Python -
Use the
repr()
Function to Convert a Set to a String in Python
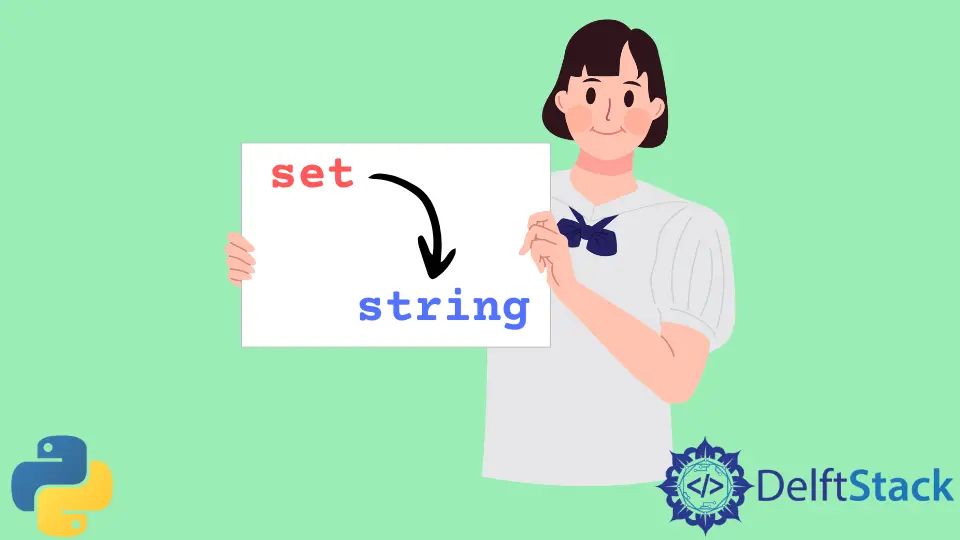
This tutorial demonstrates how to convert a Python set to a string using optimum techniques.
We can employ two techniques for the required conversion.
- Using the
map()
andjoin()
functions. - Using the
repr()
function.
Let us start by taking a sample set to work on.
se = set([1, 2, 3])
Now we have a set that we will convert into a string.
Use the map()
and join()
Functions to Convert a Set to a String in Python
In this approach, we will convert each element of a set into a string and then join them to get a single string where we will use ','
as a delimiter. We do this in the following way.
str_new = ", ".join(list(map(str, se)))
In the above code, the map()
function takes each set element and converts them into a string stored in a list using the list()
function. The join()
function then joins the individual string elements in the string with ','
as a delimiter and returns a single string.
We will now print the new string along with the data type.
print(str_new)
print(type(str_new))
The above code gives us the below output.
'1, 2, 3'
<class 'str'>
The output shows that we have converted our set into a string.
Use the repr()
Function to Convert a Set to a String in Python
This approach will convert our set into a string using the repr()
function. We will use the same sample set as in the above technique.
We will create a new variable to store the return value of the repr()
function.
r_str = repr(se)
As we can see above, we need to pass our input set to the repr()
function, and it returns the string. We will print the new variable and its data type to ensure our conversion was successful.
print(r_str)
print(type(r_str))
The above results into below output.
{1, 2, 3}
<class 'str'>
Our set was successfully converted to a string, as we can see above.
Thus we have successfully learned multiple techniques to convert Python set into a string.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python