Python Set Pop() Method
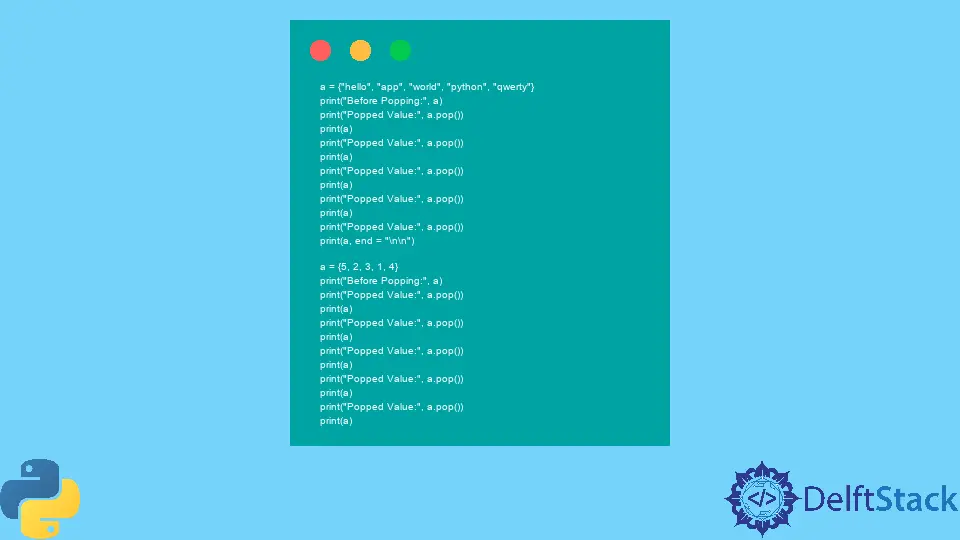
Set is an in-built data structure in Python. Elements stored inside a set are unordered and unchangeable.
Unordered means that the elements inside a set do not have a fixed order. Unchangeable means that the elements can not be changed once they are added to the set.
Additionally, a set does not allow any duplicate values. If we try to add an already existing value to a set, it will not be added.
We get the topmost element when elements are popped or removed from a set. We can perform the popping operation using Python’s pop()
method. In this article, we will learn about this method.
the pop()
Method of a Set in Python
The pop()
method pops the topmost element out of a set
. If no element exists in a set, it throws the following error.
TypeError: pop expected at least 1 arguments, got 0
Refer to the following Python code to understand how the set()
method works with the help of some relevant examples.
a = {"hello", "app", "world", "python", "qwerty"}
print("Before Popping:", a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a, end="\n\n")
a = {5, 2, 3, 1, 4}
print("Before Popping:", a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
print("Popped Value:", a.pop())
print(a)
Output:
Before Popping: {'qwerty', 'world', 'python', 'hello', 'app'}
Popped Value: qwerty
{'world', 'python', 'hello', 'app'}
Popped Value: world
{'python', 'hello', 'app'}
Popped Value: python
{'hello', 'app'}
Popped Value: hello
{'app'}
Popped Value: app
set()
Before Popping: {1, 2, 3, 4, 5}
Popped Value: 1
{2, 3, 4, 5}
Popped Value: 2
{3, 4, 5}
Popped Value: 3
{4, 5}
Popped Value: 4
{5}
Popped Value: 5
set()