How to Refresh Page in Python Selenium
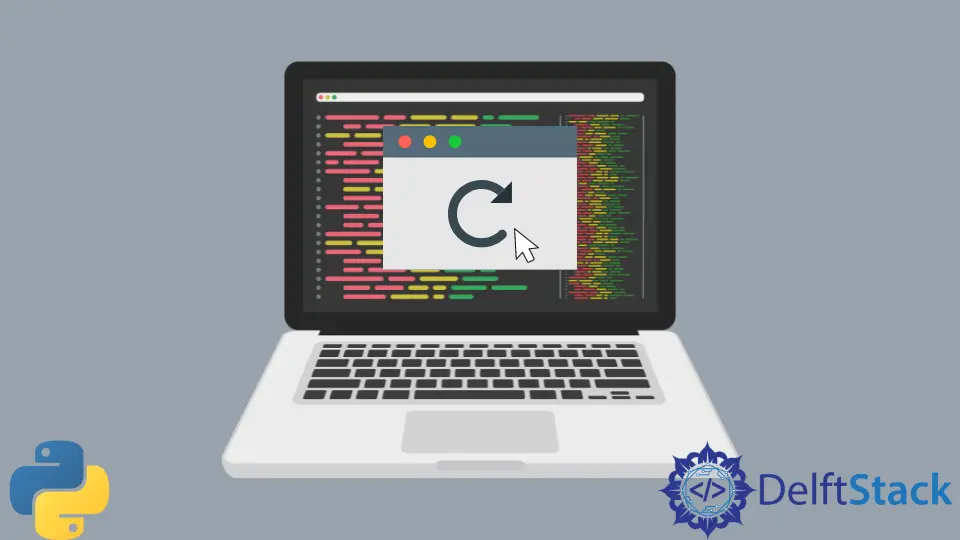
Selenium is one of the most powerful tools for web automation, and it is functional for almost all browsers and major operating systems (OS) like Windows, macOS, and Linux. In this article, we will learn how to use Selenium to refresh a webpage in Python.
Install Selenium in Python
To install Selenium for Python on your local machine, you can use the famous pip command in Command Line Interface (CLI) as follow.
pip install selenium
The above command will download and install Selenium. Once it’s done, you can import Selenium into your Python program.
Let’s check the version of Selenium to verify that it’s successfully installed on our machines.
import selenium
print(selenium.__version__)
Output:
4.4.3
Use Selenium to Refresh a Web Page
We can use Selenium to refresh a web page and do many other automated tasks, but this article focuses on using Selenium to refresh a web page in Python. First, let’s download the necessary webdrivers.
In Python, Selenium requires a driver to interact with the selected browser, and it is necessary to install the drivers so you provide its path in the code. For this article, I’ll be using Chrome as a browser and downloading the drivers for it.
driver = webdriver.Chrome(executable_path=r"D:\chromedriver.exe")
You can see in the above example snippet that I have chosen my browser as Chrome and provided it the driver’s path (executable_path=r"D:\chromedriver.exe")
.
Code Example:
from selenium import webdriver
# set the chromodriver.exe path
driver = webdriver.Chrome(executable_path=r"D:\chromedriver.exe")
# launch URL
driver.get("https://www.delftstack.com/")
# crefresh page
driver.refresh()
# close
driver.close()
Output:
The above code will pop up a new, redirecting you to the provided URL as follow.
driver.get("https://www.delftstack.com/"
Displayed a message on the header bar of Chrome saying that Chrome is being controlled by automated test software
, which is nothing but Selenium.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn