How to Run Bash Command in Python
-
Run Bash Commands in Python Using the
run()
Method of thesubprocess
Module -
Run Bash Commands in Python Using the
Popen()
Method of thesubprocess
Module
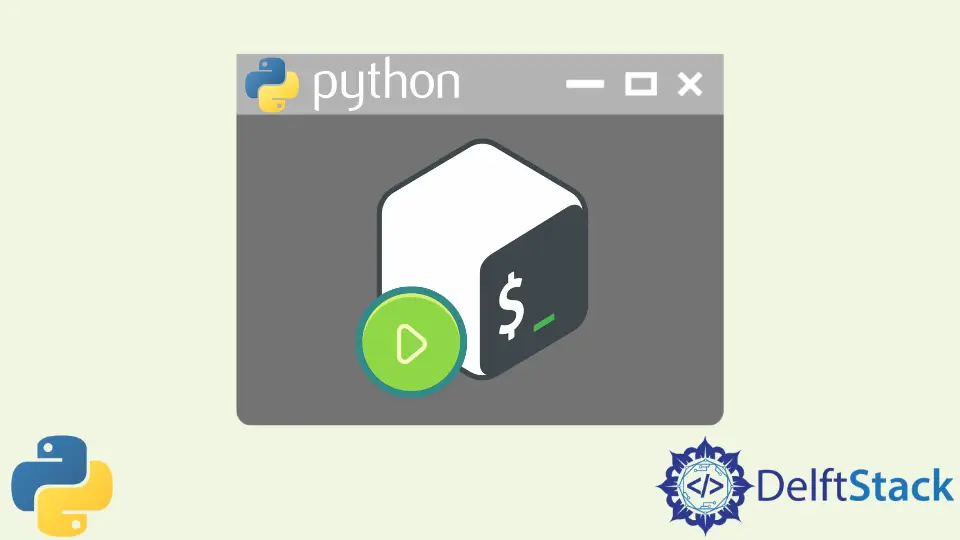
This tutorial will explain various ways to run bash commands in Python. Bash is a shell or command language interpreter used in Linux and Unix operating systems. And the bash command is an instruction from a user to the operating system to perform a specific task, like the cd
command to change the current directory, the mkd
command to make a new directory, and the ls
command to list files and subdirectories in a directory, etc.
Run Bash Commands in Python Using the run()
Method of the subprocess
Module
The run()
method of the subprocess
module takes the command passed as a string. To get output or the command’s output error, We should set the stdout
argument and the stderr
argument to PIPE
. The run
method returns a completed process containing stdout
, stderr
, and returncode
as attributes.
The code example code demonstrates how to use the run()
method to run a bash command in Python.
from subprocess import PIPE
comp_process = subprocess.run("ls", stdout=PIPE, stderr=PIPE)
print(comp_process.stdout)
Run Bash Commands in Python Using the Popen()
Method of the subprocess
Module
The Popen()
method of the subprocess
module has similar functionality as the run()
method, but it is tricky to use. The Popen()
method, unlike the run()
method, does not return a completed process object as output, and the process started by the Popen()
method needs to be handled separately, which makes it tricky to use.
Instead of the completed process, the Popen()
method returns a process object as output. The returned process needs to be killed using the process.kill()
or process.terminate()
method.
Like the run()
method, we need to set the stdout
and stderr
arguments of the Popen()
to get the command’s output and error. And the output and error can be accessed through the returned process object using the process.communicate
method.
The code example below demonstrates how to run a bash command using Popen()
method and how to get stdout
and stderr
values and then kill the process in Python:
from subprocess import PIPE
process = subprocess.Popen("ls", stdout=PIPE, stderr=PIPE)
output, error = process.communicate()
print(output)
process.kill