How to Fix Python Return Outside Function Error
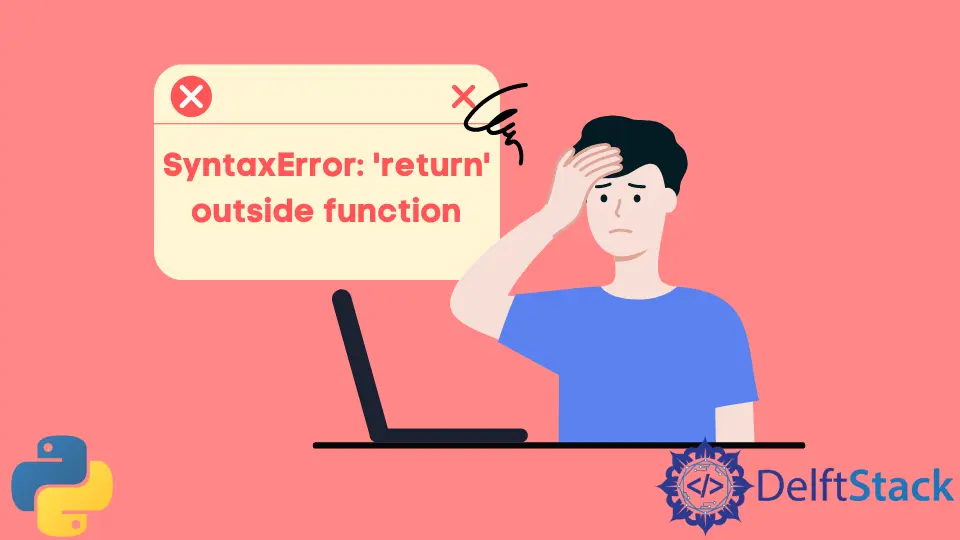
The keyword return
is reserved for functions in Python. Thus, anytime you try to use it the other way, you will get this error: return outside function
.
This compact guide is all about solving this error. Let’s dive in.
Fix return outside function
Error in Python
This error is self-explanatory; it clearly states that the return
keyword is placed outside the function. Take a look at the following code.
# Single Return statement
return i
# return inside the If
if i == 5:
return i
# Return Statement inside loop
for i in range(10):
return i
All the ways of using the return
keyword are wrong in the above code example. All these statements will give you this exact error.
The correct way of using the return
keyword is by placing it inside the function. The return
keyword is used to return a value according to the return types of functions.
As you already know that functions return some value; we use the return
keyword for this purpose. Take a look.
# Return Statment inside the function
def my_Func():
return 5
# Return Statment inside the if and function
def my_Func():
if True:
return 5
# Return Statment inside loop and Function
def my_Func():
for i in range(10):
if i == 5:
return i
As seen in the above code, all the return
statements are now placed inside a function. As such, we do not see the error anymore.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Function
- How to Exit a Function in Python
- Optional Arguments in Python
- How to Fit a Step Function in Python
- Built-In Identity Function in Python
- Arguments in the main() Function in Python
- Python Functools Partial Function
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python