Python Annotation ->
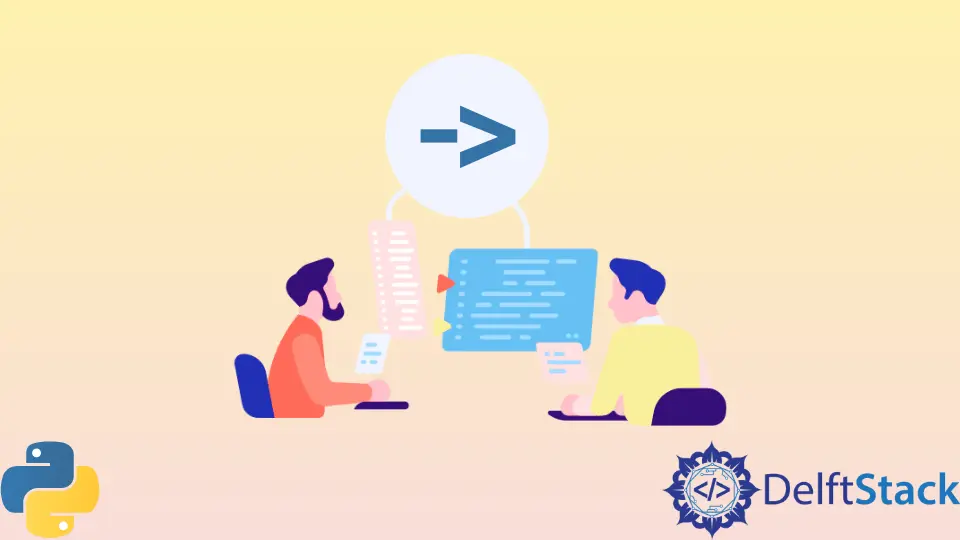
The arrow operator in python, represented by ->
, is a return value annotation, which is a part of function annotation. Function annotations are supported only in Python 3.x.
The main motive is to provide a documented code and a standard way to associate a data type hint with functioning arguments and returning value.
Function annotations are not evaluated at run time. They are considered only at compile time. These annotations are handy when using third-party libraries like mypy
. Function annotation does not define static typing to variables.
Code will not throw an exception even if the value and annotation data type does not match. Although in some IDE’s like Pycharm will show a warning if the type of value and type specified in function annotation do not match.
Annotations are only used as a hint for expected data type just for the understandability of code for developers by giving information about expected data types and return type of functions. So ->
operator annotates the type of return value.
We can print the function annotations by writing .__annotations__
with the function name, just as shown in the code below.
In this code, int is the return value annotation of the function, which is specified using ->
operator.
Example Code:
# python 3.x
def add(a, b) -> int:
return a + b
print(add(2, 3))
print(add.__annotations__)
Output:
# python 3.x
5
{'return': <class 'int'>}
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn