How to Restart Script in Python
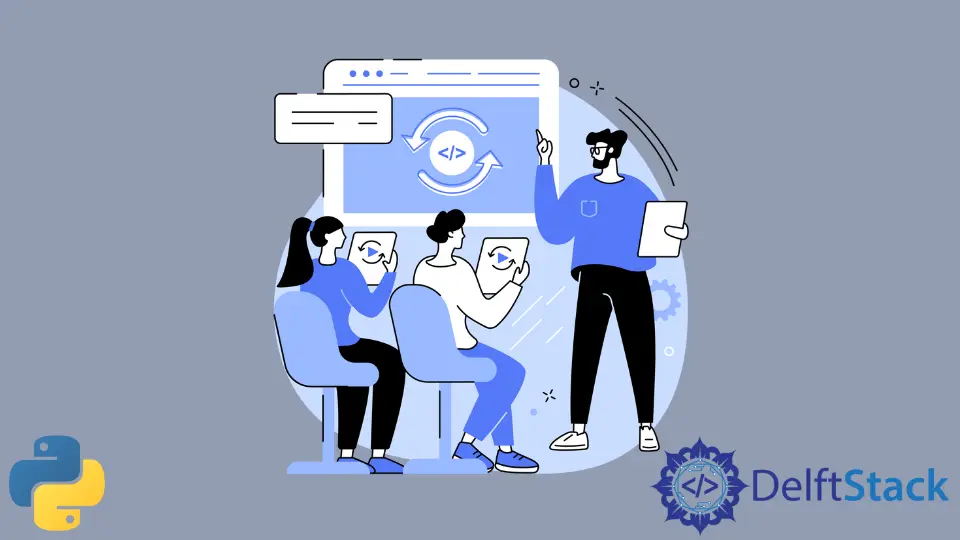
In this tutorial, we will look into the method to restart or rerun the program in Python. Suppose we want to add the functionality to our program to restart when the user chooses the restart option; we need some method to rerun the program from within the program.
This tutorial will demonstrate the method that we can use to restart the program from within the program and kill the program’s current instance in Python. We can do so by using the following methods.
Restart the Program Script in Python Using the os.execv()
Function
The os.execv(path, args)
function executes the new program by replacing the process. It does not flush the buffers, file objects, and descriptors, so the user needs to separately buffer them before calling the os.execv()
function. The os.execv()
function does not require the path
parameter to locate the executable program.
Therefore, to restart a program using the os.execv()
function, we first need to flush the buffers and file descriptors using the sys.stdout.flush()
and file.flush()
methods and then call the os.execv()
method. See the below example code.
import os
import sys
sys.stdout.flush()
os.execv(sys.argv[0], sys.argv)