How to Query String With Params for Requests in Python
- Understanding Query Strings
- Using the Requests Library
- Handling Special Characters
- Sending POST Requests with Query Parameters
- Conclusion
- FAQ
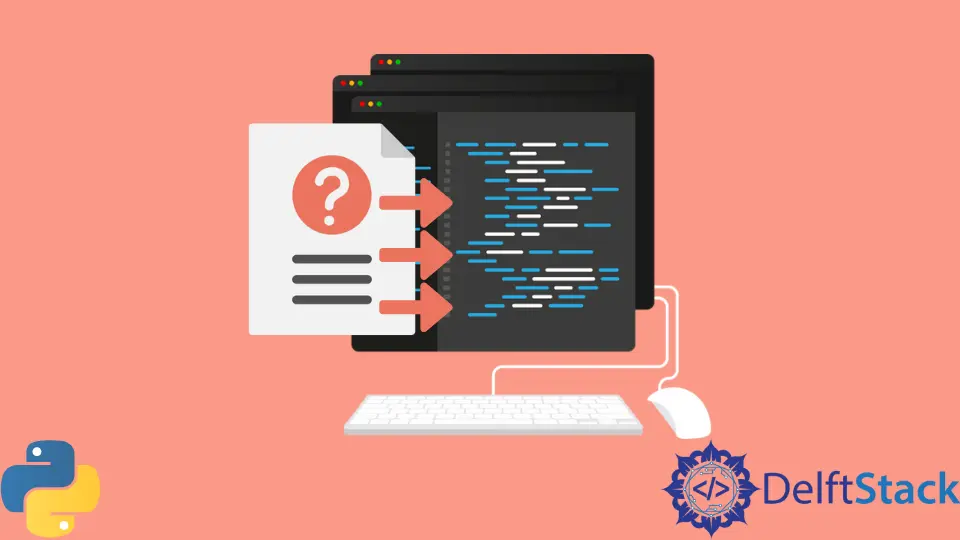
In the world of web development, interacting with APIs is a common task. One of the most crucial components of making API requests is understanding how to construct query strings with parameters.
This tutorial will guide you through the process of providing query strings with parameters for requests in Python. Whether you’re fetching data from a public API or sending information to a server, knowing how to format your requests correctly is essential. We’ll explore various methods to achieve this, complete with practical examples and explanations. So, let’s dive in and make your Python requests more effective!
Understanding Query Strings
Before we jump into the code, it’s important to understand what a query string is. A query string is a part of a URL that assigns values to specified parameters. It usually starts with a question mark (?) and can contain one or more parameters separated by ampersands (&). For example, in the URL https://api.example.com/data?param1=value1¶m2=value2
, param1
and param2
are the parameters, and value1
and value2
are their respective values.
When making requests in Python, particularly using the requests
library, you can easily include these parameters to tailor your API calls. Let’s explore how to do this effectively.
Using the Requests Library
The requests
library in Python is a powerful tool for making HTTP requests. It simplifies the process of sending requests and handling responses. To include query string parameters, you can use the params
argument in the get
method. Here’s how it works:
import requests
url = 'https://api.example.com/data'
params = {
'param1': 'value1',
'param2': 'value2'
}
response = requests.get(url, params=params)
print(response.url)
Output:
https://api.example.com/data?param1=value1¶m2=value2
In this example, we first import the requests
library. We define the base URL of the API we want to interact with. The params
dictionary holds our query parameters. When we call requests.get()
, we pass the params
dictionary to the params
argument. The library automatically constructs the full URL with the query string, which we can verify by printing response.url
. This approach is clean and efficient, allowing you to easily modify your parameters without manually constructing the query string.
Handling Special Characters
When working with query strings, you might encounter special characters that need to be encoded properly. The requests
library automatically handles this for you, but it’s good to know how to encode parameters manually when necessary. Here’s an example of how to do this using the urllib.parse
module:
import requests
from urllib.parse import urlencode
url = 'https://api.example.com/data'
params = {
'param1': 'value with spaces',
'param2': 'value&with&special#characters'
}
encoded_params = urlencode(params)
response = requests.get(f"{url}?{encoded_params}")
print(response.url)
Output:
https://api.example.com/data?param1=value+with+spaces¶m2=value%26with%26special%23characters
In this code, we first import the urlencode
function from the urllib.parse
module. We then define our parameters, which include spaces and special characters. By using urlencode
, we convert our parameters into a properly encoded query string. Finally, we concatenate this encoded string to our URL and make the GET request. This method ensures that your parameters are correctly formatted, preventing errors when interacting with APIs.
Sending POST Requests with Query Parameters
While GET requests are commonly used for fetching data, you may also need to send parameters with POST requests. This is often done when submitting forms or sending data to an API. You can still include query parameters in a POST request using the same params
argument. Here’s an example:
import requests
url = 'https://api.example.com/submit'
params = {
'param1': 'value1',
'param2': 'value2'
}
data = {
'field1': 'data1',
'field2': 'data2'
}
response = requests.post(url, params=params, data=data)
print(response.url)
Output:
https://api.example.com/submit?param1=value1¶m2=value2
In this example, we define a new URL for a POST request. We still create our parameters in the params
dictionary, but we also have a data
dictionary to hold the body of the request. When we call requests.post()
, we pass both params
and data
. The requests
library constructs the full URL with the query parameters and sends the data in the body of the request. This flexibility allows you to send additional data while still including query parameters.
Conclusion
Mastering how to query strings with parameters in Python is essential for effective API interaction. The requests
library simplifies this process, allowing you to send GET and POST requests effortlessly. By using dictionaries for parameters and understanding how to encode special characters, you can ensure that your requests are well-formed. Whether you’re fetching data or submitting forms, these techniques will enhance your skills as a Python developer. Keep practicing, and soon you’ll be comfortable handling any API request that comes your way!
FAQ
-
How do I install the requests library in Python?
You can install the requests library using pip by running the command pip install requests in your terminal or command prompt. -
Can I send both query parameters and body data in a POST request?
Yes, you can send both query parameters using the params argument and body data using the data argument in a POST request. -
What happens if I don’t encode special characters in query parameters?
If you don’t encode special characters, it may result in errors or unexpected behavior when making requests to an API. -
Is it possible to include headers in my requests?
Yes, you can include headers in your requests by using the headers argument in the get or post methods. -
How can I check the response status code from an API request?
You can check the response status code by accessing the status_code attribute of the response object, like response.status_code.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn