Python __repr__ Method
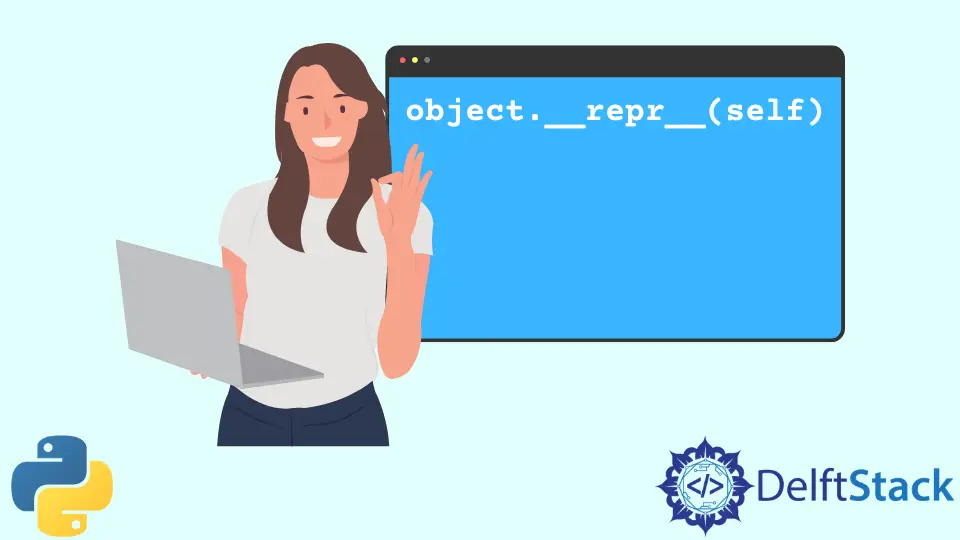
This article introduces the __repr__
method in Python.
The __repr__
method is a special method used inside a class that represents an object of a class in the form of a string. The in-built repr()
function is utilized to call the __repr__
method.
The __repr__
method can simply be utilized to produce and define your own string representation of the objects of a class. This method is mainly utilized for debugging in Python.
The syntax for the __repr__
method is as follows:
object.__repr__(self)
We use the keyword self
here as the __repr__
method is a special method utilized in enriching classes, and the self
keyword represents the instance of the class involved.
The developers tend to generally favor the __repr__
method as it is unambiguous, while the end-users utilize the __str__
method because it is easier to read and understand.
This method can simply get called by the in-built repr()
function. Here is an example code that will help make things clearer.
class Employee:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
rep = "Employee(" + self.name + "," + str(self.age) + ")"
return rep
emp = Employee("Archie", 19)
print(repr(emp))
The above code provides the following output:
Employee(Archie,19)
As you can see, the function repr()
takes in a single parameter, the object of the class.
The repr()
function generally only provides the real-time string representation of an object of the class, but it can be overridden with the help of the __repr__
method to make it work differently in a way that the user wants.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn