How to Replace Newline With Space in Python
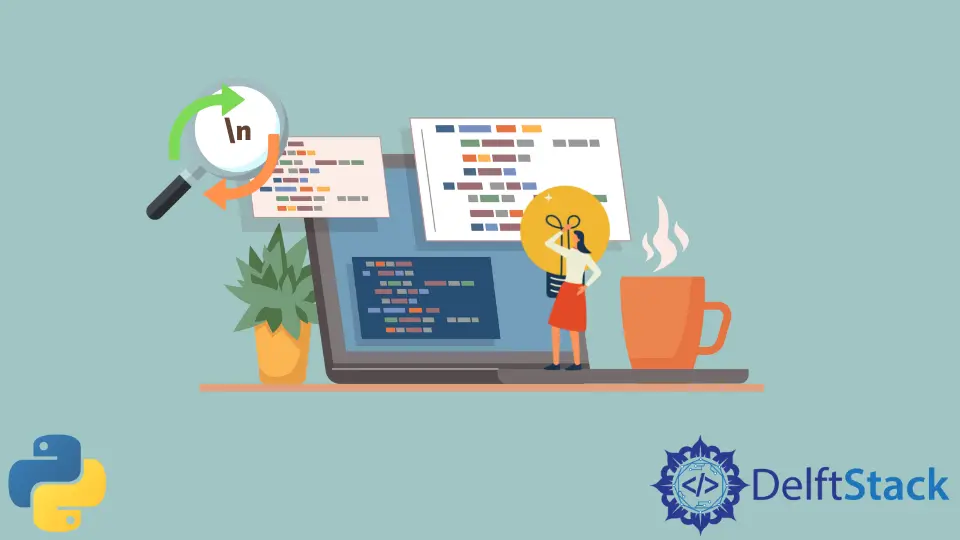
In this tutorial, we will learn to employ a technique to replace newlines with spaces in Python.
Use the replace()
Function to Replace Newline With Space in Python
Let us take a sample string with a new line character to work with.
string1 = "Hello\nWorld"
Now we have a string with a new line character. Let us print our sample string.
print(string1)
The above code displays the below output demonstrating the new line.
Hello
World
We will now use the replace()
function to replace our newline character with space. We pass our string as a parameter to the function and store the new resultant string in a new variable.
string2 = string1.replace("\n", " ")
The above code will replace all the newline characters in our string with spaces. We get a string with no line characters in it.
We will now print the new string to see the output.
print(string2)
We get the below output on printing the new string.
Hello World
We can see that a space has been added in place of a newline character.
Thus, we can conveniently replace newline characters with space in Python with the above method.