How to Remove List From List in Python
-
Remove List B From List a Using the
remove()
Method in Python -
Remove List B From List a Using the
difference()
Method in Python
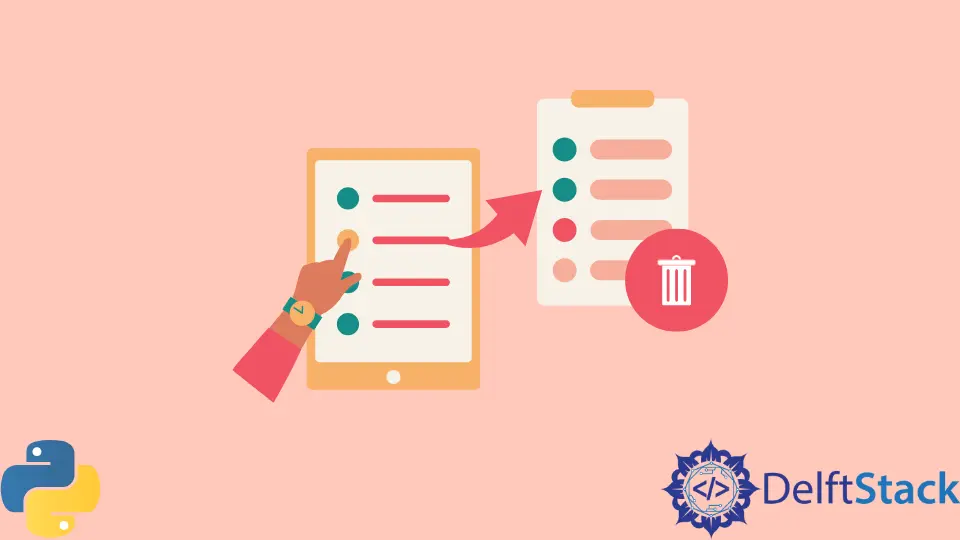
The list in Python is a data structure that contains an order sequence of items. We can perform many operations on the list. Let’s say we want to remove a list B from list A. It simply means that we want to remove the items from list A that are also present in list B.
For Example we have a list A which contains items ["Blue", "Pink", "Purple", "Red"]
and List B contains the items ["Silver", "Red", "Golden", "Pink"]
. Now, if we remove list B from list A, in the output, we will get list A as ["Blue", "Purple"]
because these items were also present in list B. We can do this task either by using the remove()
function with a list or using the difference()
function available with the set
data structure.
Remove List B From List a Using the remove()
Method in Python
In this example, we will use the remove()
method on list A to remove the items that are similar in list A and list B. We use the remove()
method with list A so that items will be removed from list A, but list B will be the same as before. In this code, we iterate over the items of list A and check if that item is also present in list B; the item will be removed from list A.
Example Code:
# Python 3.x
list_A = ["Blue", "Pink", "Purple", "Red"]
list_B = ["Silver", "Red", "Golden", "Pink"]
print("List A before:", list_A)
print("List B before:", list_B)
for item in list_A:
if item in list_B:
list_A.remove(item)
print("List A now:", list_A)
print("List B now:", list_B)
Output:
List A before: ['Blue', 'Pink', 'Purple', 'Red']
List B before: ['Silver', 'Red', 'Golden', 'Pink']
List A now: ['Blue', 'Purple']
List B now: ['Silver', 'Red', 'Golden', 'Pink']
Remove List B From List a Using the difference()
Method in Python
Another way to remove similar items from list A is to subtract them from list B. With the set
data structure, there is a method difference()
that will return the items present in set A but not in set B. It returns only the different items of set A, which are unique between the two sets. But as this method is available with set
.
So in our code, we will first cast both lists to set, then apply the set_A.difference(set_B)
function, and we will store the result again in list_A by casting the result to list data type.
Example Code:
# Python 3.x
list_A = ["Blue", "Pink", "Purple", "Red"]
list_B = ["Silver", "Red", "Golden", "Pink"]
print("List A before:", list_A)
print("List B before:", list_B)
setA = set(list_A)
setB = set(list_B)
list_A = list(setA.difference(list_B))
print("List A now:", list_A)
print("List B now:", list_B)
Output:
List A before: ['Blue', 'Pink', 'Purple', 'Red']
List B before: ['Silver', 'Red', 'Golden', 'Pink']
List A now: ['Purple', 'Blue']
List B now: ['Silver', 'Red', 'Golden', 'Pink']
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python