Regex replace() Method in Python
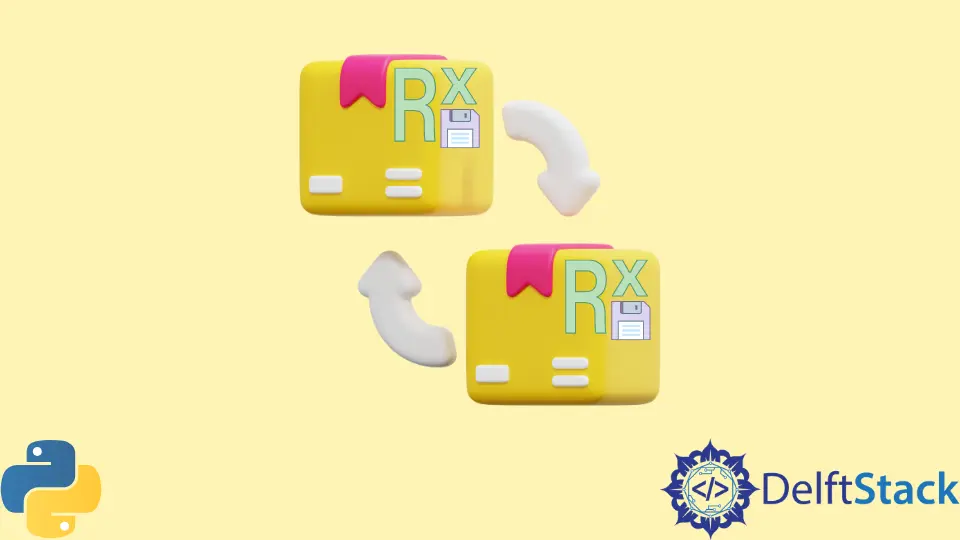
In this tutorial, we will look into the use and function of the re.sub()
method and look into the example codes. The Python’s re
module provides the various functionalities using regular expression for both Unicode and 8-bit strings. The functionalities include string replacement, splitting, and matching, etc.
Regex Replace Using the re.sub()
Method in Python
The re.sub(pattern, repl, string, count=0)
method takes the string
as input and replaces the leftmost occurrences of the pattern
with the repl
. If no pattern
is found in the string
argument, the string
is returned without any changes.
The pattern
argument must be in the form of a regular expression. The repl
can be a string or a function. If the repl
argument is a string, then the pattern
in the string
is replaced by the repl
string. If a function is passed as the repl
argument, then the function will be called whenever an occurrence of the pattern
is found. The function takes the matchObject
as input and returns the replacement string. The matchObject
will have its value equal to True
if the match is found and will have its value equal to None
otherwise.
The optional count
argument represents the maximum occurrences of the pattern
we want to replace in the string
.
The below example code demonstrates how to use the re.sub()
method to replace some pattern from the string using the regular expression:
import re
string = "Hello! How are you?! Where have you been?!"
new_string = re.sub(r"""[!?'".<>(){}@%&*/[/]""", " ", string)
print(new_string)
Output:
Hello How are you Where have you been
The above code example removes the characters specified in the pattern
argument with the blank space, which is our repl
argument in the above code.
We can also use the function as the repl
argument to perform the same task by returning " "
whenever an occurrence of the repl
argument is found, as shown in the below example code:
import re
def repl_func(match):
if match == True:
return " "
string = "Hello! How are you?! Where have you been?!"
new_string = re.sub(r"""[!?'".<>(){}@%&*/[/]""", repl_func, string)
print(new_string)
Output:
Hello How are you Where have you been