Python Regex group() Function
- What is the group() Function?
-
Using the
group()
Function to Extract Substrings - Accessing All Groups with the group() Function
- Named Groups for Better Readability
- Conclusion
- FAQ
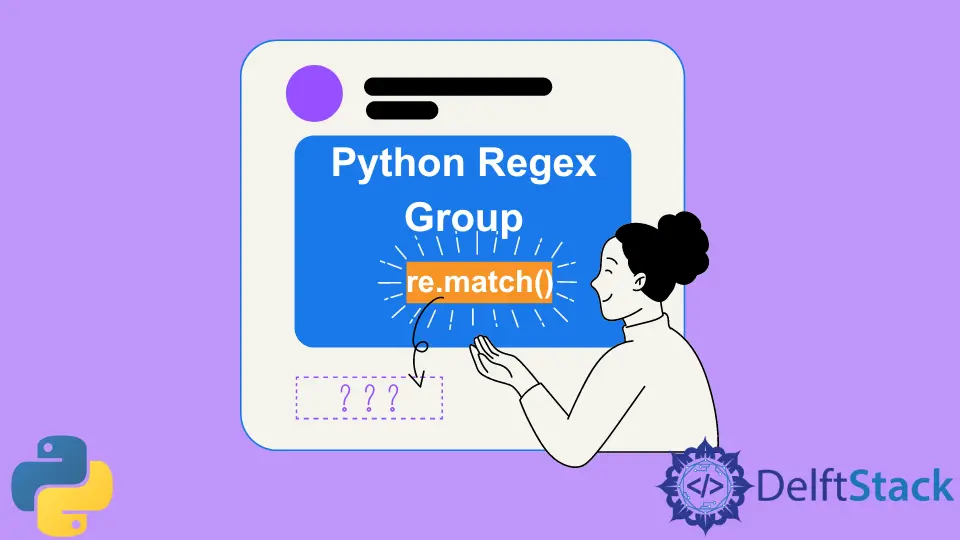
Regular expressions, or regex, are an essential tool in programming, allowing you to search, match, and manipulate strings with precision. In Python, the re
module provides robust support for regex operations, and one of its most powerful features is the group()
function. This function allows you to extract specific parts of a match, making it invaluable for data extraction, validation, and transformation tasks.
In this tutorial, we will delve into the group()
function, exploring its syntax, usage, and practical examples. By the end of this article, you’ll have a solid understanding of how to implement the group()
function in Python, enabling you to harness the full potential of regular expressions in your projects.
What is the group() Function?
The group()
function in Python’s re
module is used to retrieve specific parts of a matched string. When you apply a regex pattern to a string, the findall()
or search()
methods return a match object. This object contains various methods, including group()
, which allows you to access the captured groups from the regex pattern.
The syntax of the group()
function is straightforward:
match_object.group([group1, ...])
Here, group1
refers to the specific group you want to extract. If no argument is provided, it returns the entire match. Groups in regex are defined using parentheses ()
, allowing you to capture specific portions of the pattern.
Using the group()
Function to Extract Substrings
One common use of the group()
function is to extract substrings from a match. Let’s consider an example where we want to extract the area code and the local number from a phone number formatted as (123) 456-7890
.
import re
pattern = r'\((\d{3})\) (\d{3})-(\d{4})'
phone_number = '(123) 456-7890'
match = re.search(pattern, phone_number)
if match:
area_code = match.group(1)
local_number = match.group(2)
print(f'Area Code: {area_code}')
print(f'Local Number: {local_number}')
Output:
Area Code: 123
Local Number: 456
In this example, we define a regex pattern that captures the area code and the local number from a phone number. The parentheses in the pattern create groups, allowing us to extract them using match.group(1)
for the area code and match.group(2)
for the local number. This method is particularly useful when dealing with structured data formats, making it easy to retrieve specific components.
Accessing All Groups with the group() Function
Sometimes, you may want to access all the captured groups at once. The group()
function can take an integer argument or a tuple of integers to return multiple groups. Let’s modify our previous example to retrieve all parts of the phone number in one go.
import re
pattern = r'\((\d{3})\) (\d{3})-(\d{4})'
phone_number = '(123) 456-7890'
match = re.search(pattern, phone_number)
if match:
all_groups = match.groups()
print(f'All Groups: {all_groups}')
Output:
All Groups: ('123', '456', '7890')
Here, we use match.groups()
to retrieve all captured groups as a tuple. This is particularly useful when you need to handle multiple pieces of information at once, allowing for more streamlined data processing. The groups()
method returns a tuple containing all the captured groups, which can then be easily manipulated or displayed as needed.
Named Groups for Better Readability
Using numbered groups can sometimes lead to confusion, especially in more complex regex patterns. Python allows you to use named groups, which can enhance the readability of your code. Named groups are defined using the syntax (?P<name>...)
. Let’s look at an example.
import re
pattern = r'(?P<area_code>\d{3})-(?P<local_number>\d{3})-(?P<line_number>\d{4})'
phone_number = '123-456-7890'
match = re.search(pattern, phone_number)
if match:
area_code = match.group('area_code')
local_number = match.group('local_number')
line_number = match.group('line_number')
print(f'Area Code: {area_code}')
print(f'Local Number: {local_number}')
print(f'Line Number: {line_number}')
Output:
Area Code: 123
Local Number: 456
Line Number: 7890
In this example, we define named groups for the area code, local number, and line number. By using match.group('area_code')
, we can access the specific parts of the phone number in a more intuitive manner. This approach not only makes the code easier to read but also reduces the risk of errors when working with multiple groups.
Conclusion
The group()
function in Python’s regex module is a powerful tool for extracting specific parts of a match. Whether you’re dealing with structured data or simply need to validate input formats, understanding how to effectively use group()
can significantly enhance your programming capabilities. From extracting substrings to utilizing named groups for better readability, the group()
function provides the flexibility needed for efficient string manipulation. With the examples and explanations provided in this tutorial, you’re now equipped to implement regex in your Python projects confidently.
FAQ
-
What does the group() function do in Python?
The group() function retrieves specific parts of a match from a regex pattern. -
How do I access multiple groups using the group() function?
You can access multiple groups using match.groups() to get all captured groups as a tuple. -
Can I use named groups in regex patterns?
Yes, named groups can be created using the syntax (?P…) for better readability. -
What is the difference between group() and groups()?
group() retrieves a specific group by index or name, while groups() returns all captured groups as a tuple. -
How can I improve regex readability in Python?
Using named groups in your regex patterns can significantly enhance readability and reduce confusion.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn