How to Solve Reduce Is Not Defined in Python
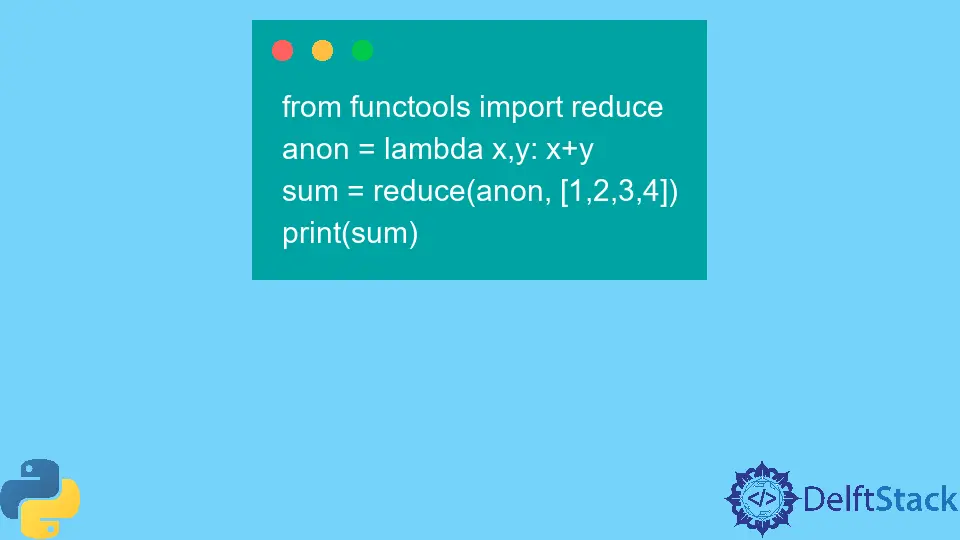
From time to time, we often face Not Defined
error messages and, more broadly, NameError
. These error messages are typical and easy to solve if a simple understanding of what has been named within your Python code exists.
The NameError
and Not Defined
errors stem from a non-existent variable, binding, function, or library. For this context, the reduce
function does not exist within your code.
This article will discuss solving the reduce is not defined
error within your Python code.
Use functools
to Solve NameError: name 'reduce' is not defined
in Python
The reduce()
function helps compute a single value from a list using a lambda function on each element within the list. The reduce()
function builds a value by taking the current element from the array and combining or comparing it to the current value until it goes through all the elements within the list.
Pre-Python 3, the reduce()
function worked as a built-in function; therefore, the below code snippet to sum up all the elements within a list would have worked.
def anon(x, y):
return x + y
sum = reduce(anon, [1, 2, 3, 4])
print(sum)
However, if you run the above code, it would give the below error:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\HTML\python\txt.py", line 2, in <module>
l = reduce(anon, [1,2,3,4])
NameError: name 'reduce' is not defined
That is because reduce()
is no longer a built-in function but a function within a built-in library called functools
, which contains higher-order functions and operations on callable objects. As reduce()
is a higher-order function, it makes sense to be present.
reduce()
is a higher-order function because it takes as its argument another function. Now to make use of reduce()
, we need to import functools
:
import functools
def anon(x, y):
return x + y
sum = functools.reduce(anon, [1, 2, 3, 4])
print(sum)
The output of the code:
10
We can make it easier within our code to use the from
keyword to import reduce
:
from functools import reduce
def anon(x, y):
return x + y
sum = reduce(anon, [1, 2, 3, 4])
print(sum)
The output of the code:
10
We no longer have a reduce is not defined
error within our code and can now understand what to look for when we see such.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python