How to Fix Python Recursionerror: Maximum Recursion Depth Exceeded in Comparison Error
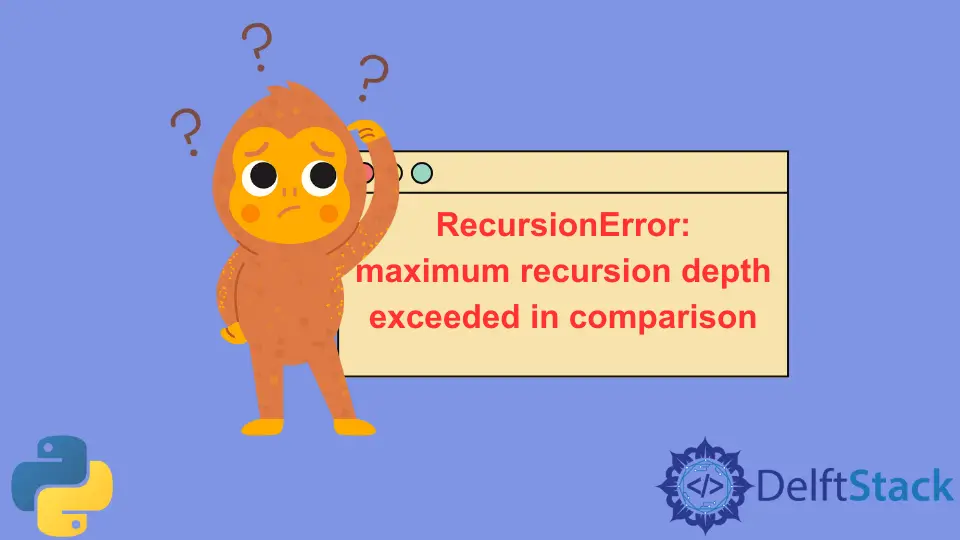
This article will introduce how you can solve recursionerror: maximum recursion depth exceeded in comparison
error in Python. First, we need to understand what recursion is in the programming language.
Fix recursionerror: maximum recursion depth exceeded in comparison
Error in Python
Recursion occurs when you call the function itself inside its body. It works like a loop until a certain condition is met; the recursion continues. But the case is different in Python. There is a maximum recursion depth limit in Python. For instance, take a look at the following code example.
# function definition
def func():
print("Hello Python")
# recursive Call
func()
# uncomment this to run the function
# func()
If you run the above code, it will print the Hello Python
until a certain limit; then, it will give this exact error. So, how can you adjust the limit according to your choice? You can import a module and check for the maximum recursion depth. Take a look at the following code.
# import module
import sys
# function to check the default maximum recursion depth
print(sys.getrecursionlimit())
By running the above code, you will get the recursive limit of your system. You can check the maximum recursion depth using the above code. To adjust the limit, you can run the following code.
# To increase or decrease the limit
sys.setrecursionlimit(2000)
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python