How to Read XLSX Files Using Python and Pandas
- What is Pandas and Why Use It?
- Setting Up Your Environment
- Reading XLSX Files with Pandas
- Specifying Sheet Names
- Reading Specific Columns
- Handling Missing Values
- Conclusion
- FAQ
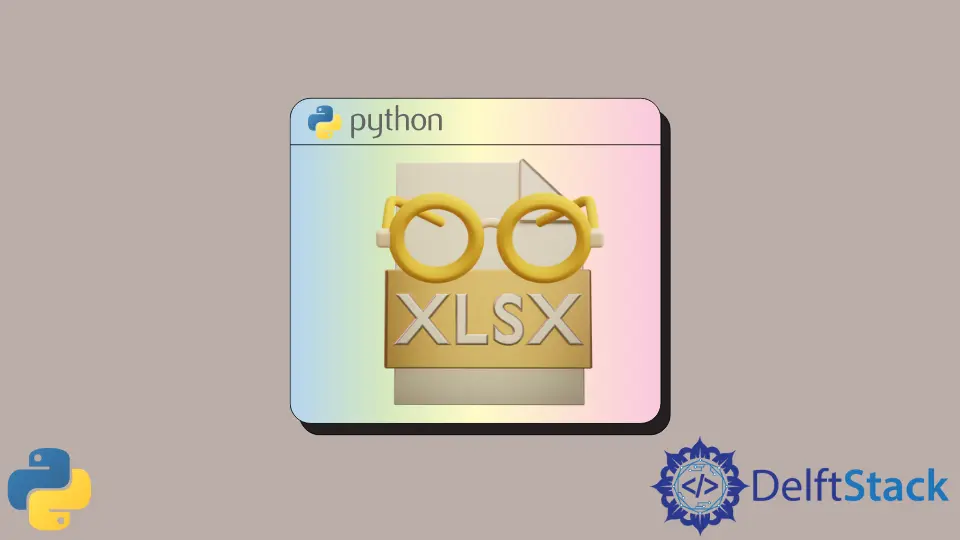
In today’s data-driven world, working with spreadsheets is a common task for many professionals. XLSX files, which are Microsoft Excel’s modern file format, are widely used for storing data in a structured way. If you’re a Python enthusiast or a data analyst, knowing how to read XLSX files using the Pandas library can significantly streamline your data handling processes.
This article will guide you through the steps to read .xlsx files effortlessly with Python and Pandas. By the end, you’ll be equipped with the knowledge to manipulate and analyze data stored in Excel spreadsheets, making your data analysis tasks much more efficient.
What is Pandas and Why Use It?
Pandas is a powerful data manipulation and analysis library for Python. It provides data structures like DataFrames and Series that make it easy to work with structured data. One of the standout features of Pandas is its ability to read and write various file formats, including Excel files. This capability is essential for data analysts who frequently deal with Excel spreadsheets.
By using Pandas, you can quickly import data from .xlsx files into a DataFrame, which allows for efficient data manipulation, analysis, and visualization. Whether you’re working on a small project or a large-scale data analysis task, Pandas simplifies the process of handling Excel files.
Setting Up Your Environment
Before diving into reading .xlsx files, ensure that you have the necessary libraries installed. You can easily install Pandas and openpyxl, which is required for reading Excel files, using pip. Open your terminal or command prompt and run the following commands:
pip install pandas openpyxl
Installing these libraries is crucial, as they provide the functionality needed to read Excel files in Python. Once installed, you can start working with your .xlsx files right away.
Reading XLSX Files with Pandas
Now that your environment is set up, let’s explore how to read .xlsx files using Pandas. The primary function used for this task is pd.read_excel()
, which allows you to load an Excel file into a DataFrame. Below is a simple example:
import pandas as pd
file_path = 'data.xlsx'
df = pd.read_excel(file_path)
print(df.head())
In this code snippet, we import the Pandas library and specify the path to our .xlsx file. The pd.read_excel()
function reads the file and loads it into a DataFrame called df
. Finally, we use the print()
function to display the first five rows of the DataFrame.
Output:
Column1 Column2 Column3
0 1 4 7
1 2 5 8
2 3 6 9
This output shows the first five rows of the DataFrame, giving you a quick overview of your data. You can easily manipulate this DataFrame using various Pandas functions to analyze or visualize your data further.
Specifying Sheet Names
In many cases, an Excel file contains multiple sheets. You can specify which sheet to read by using the sheet_name
parameter in the pd.read_excel()
function. Here’s how you can do that:
df_sheet = pd.read_excel(file_path, sheet_name='Sheet1')
print(df_sheet.head())
In this example, we specify the sheet name as ‘Sheet1’. If you have multiple sheets in your Excel file, you can either provide the sheet name as a string or the sheet index as an integer (0 for the first sheet, 1 for the second, and so forth).
Output:
Column1 Column2 Column3
0 1 4 7
1 2 5 8
2 3 6 9
By specifying the sheet name, you can focus on the data that is most relevant to your analysis. This flexibility allows you to work with complex Excel files seamlessly.
Reading Specific Columns
Sometimes, you might only need specific columns from your Excel file. You can achieve this by using the usecols
parameter in the pd.read_excel()
function. Here’s an example:
df_columns = pd.read_excel(file_path, usecols=['Column1', 'Column3'])
print(df_columns.head())
In this code snippet, we specify the columns we want to read: ‘Column1’ and ‘Column3’. This approach can save memory and processing time, especially when dealing with large datasets.
Output:
Column1 Column3
0 1 7
1 2 8
2 3 9
Reading only the necessary columns not only optimizes performance but also simplifies your DataFrame, making it easier to work with.
Handling Missing Values
When working with real-world data, missing values are a common occurrence. Pandas provides several methods to handle these missing values. You can specify how to handle them while reading the Excel file using the na_values
parameter. Here’s an example:
df_missing = pd.read_excel(file_path, na_values=['NA', 'NULL', ''])
print(df_missing.head())
In this example, we tell Pandas to treat ‘NA’, ‘NULL’, and empty strings as missing values. This way, you can ensure that your DataFrame accurately reflects the data’s integrity.
Output:
Column1 Column2 Column3
0 1 4 7
1 2 5 8
2 3 6 NaN
By effectively managing missing values, you can improve the quality of your data analysis and avoid potential pitfalls in your results.
Conclusion
Reading XLSX files using Python and Pandas is a straightforward process that can significantly enhance your data analysis capabilities. With just a few lines of code, you can load, manipulate, and analyze data from Excel spreadsheets. Whether you are working with single sheets or multiple sheets, Pandas provides the flexibility and functionality you need to handle your data effectively. By mastering these techniques, you will be well on your way to becoming a proficient data analyst.
FAQ
- what is Pandas?
Pandas is a Python library used for data manipulation and analysis, offering data structures like DataFrames and Series.
-
how do I install Pandas and openpyxl?
You can install them using pip with the command: pip install pandas openpyxl. -
can I read multiple sheets from an Excel file?
Yes, you can specify the sheet name or index using thesheet_name
parameter in thepd.read_excel()
function. -
how can I handle missing values while reading Excel files?
You can use thena_values
parameter to specify which values should be considered as missing. -
is it possible to read only specific columns from an Excel file?
Yes, you can use theusecols
parameter to specify which columns you want to read.