How to Read a Text File Into a List in Python
-
Read a Text File to List in Python Using
read().split()
on File Object Returned byopen()
Function -
Read a Text File to List in Python Using
loadtxt
Function ofNumPy
Library -
Read a Text File to List in Python Using
csv.reader()
Function
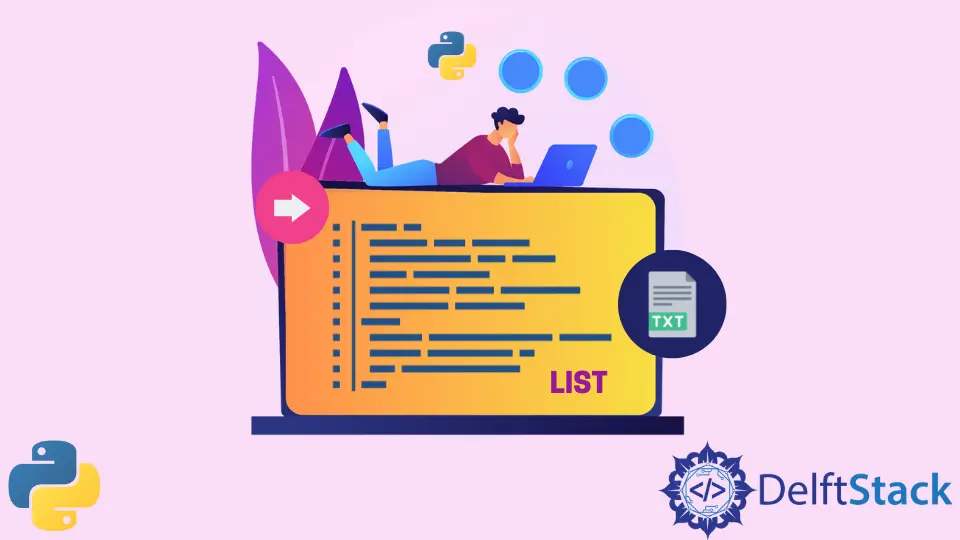
This tutorial will look into multiple methods to load or read a text file into a Python list. It includes using the read().split()
function on file object returned by the open()
function, the loadtxt
function of NumPy
library, and csv.reader
function to load a text file and divide it into separate elements in the list.
Read a Text File to List in Python Using read().split()
on File Object Returned by open()
Function
Code example given below shows how we can first read a text file using open
and then split it into an array using read().split()
functions with ,
as the delimiter.
Suppose the content of the text file file.txt
is below.
1,2,321,355,313
Code:
with open("file.txt", "r") as tf:
lines = tf.read().split(",")
for line in lines:
print(line)
Output:
1
2
321
355
313
The argument in the split()
function, ,
in the example, specifies the delimiter in the text file.
Read a Text File to List in Python Using loadtxt
Function of NumPy
Library
Code example given below shows how we can use the loadtxt
function of the NumPy
library to load and split the text file into an array using the delimiter
parameter.
from numpy import loadtxt
lines = loadtxt("file.txt", delimiter=",")
for line in lines:
print(line)
Output:
1.0
2.0
321.0
355.0
313.0
Read a Text File to List in Python Using csv.reader()
Function
csv
module is typically used to process the CSV file but could also be used to process the text file.
The reader
function of the csv
module reads the given file and returns a _csv.reader
object. We can convert the _csv.reader
object to the list by applying the list()
function.
Be aware that the converted list is a 2D array even if the file has only one line; therefore, we need to get the 1D list using the index [0]
.
import csv
with open("file.txt") as f:
line = csv.reader(f, delimiter=",")
print(list(line)[0])
Output:
['1', '2', '321', '355', '313']
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python