Raw String in Python
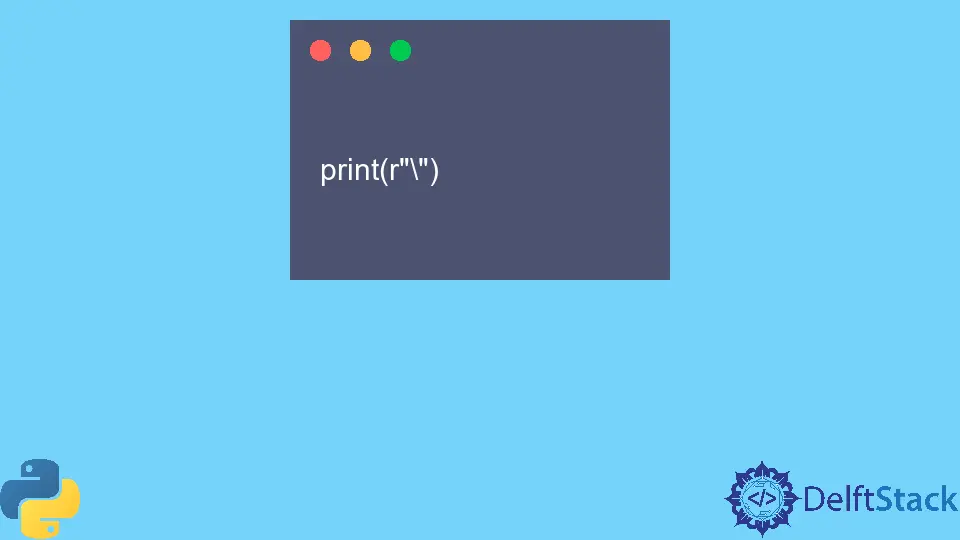
There are many ways of representing strings in Python. One way of representing strings is to convert them into raw strings.
This tutorial will define a raw string in Python.
Raw String in Python
The raw string in Python is just any usual string prefixed by an r
or R
. Any backslash (\)
present in the string is treated like a real or literal character. For example, if a string has \n
or \t
in between, it will be considered a character and not a newline
or a tab
character.
Let us take an example of using the newline \n
character in between a string without prefixing the string with r
or R
.
print("Hi\nHow are you?")
Output:
Hi
How are you?
Now let us prefix the whole string with the raw string character r
.
print(r"Hi\nHow are you?")
Output:
Hi\nHow are you?
As you can see, the newline character \n
is treated as a literal string and not some special character.
Invalid Raw Strings in Python
A single backslash \
is not considered a valid raw string in Python.
print(r"\")
Output:
File "<ipython-input-6-6cdee2fbdda0>", line 1
print(r"\")
^
SyntaxError: EOL while scanning string literal
Use of Raw Strings in Python
In Python, raw strings are used to return a string when it is not processed at all. It means if a string is prefixed with an r
or a raw string
and that string consists of any invalid escape character like \x
, then an error won’t occur.
Here’s an example code.
print("Hi\xHow are you?")
Output:
File "<ipython-input-15-1056651b28e1>", line 1
print("Hi \x How are you?")
^
SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 3-4: truncated \xXX escape
Note that the string is not prefixed with r
, and there is an invalid escape character in between the string. Hence, an error has occurred.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn