Python Quine
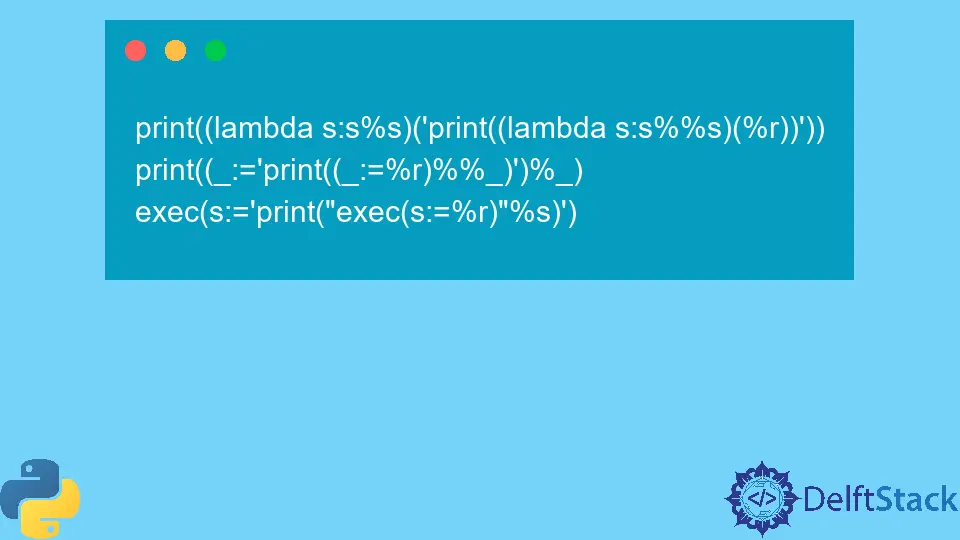
A Quine is a computer program that produces its source code as its output. The program takes no input and outputs a copy of its source code.
Quines are interesting in that they seem to defy the very purpose of programming, which is to produce output based on input. Quines can be helpful in certain situations, such as when you need to generate a copy of your program’s source code.
Run Python Quine
Creating a Quine is not particularly difficult, but it does require a bit of thought. The basic idea is to use the programming language’s built-in functionality to output the program’s source code.
In Python, you can use the built-in repr
function to output the source code of a given object.
Of course, simply calling repr
on the program’s source code will not produce a valid Quine, as the output will also include the repr
function itself. To get around this, you can use a bit of trickery to create a self-referencing string that doesn’t include the repr
process.
Once you have a self-referencing string, you can print it out to produce a valid Quine, and that’s all there is to it! You can create a program with a copy of its source code with just a short code.
Code Example:
print((lambda s: s % s)("print((lambda s:s%%s)(%r))"))
print((_ := "print((_:=%r)%%_)") % _)
exec(s := 'print("exec(s:=%r)"%s)')
Output:
print((lambda s:s%s)('print((lambda s:s%%s)(%r))'))
print((_:='print((_:=%r)%%_)')%_)
exec(s:='print("exec(s:=%r)"%s)')
Conclusion
A Quine is a program used to create outputs of its source code. The name comes from the word “quote”, meaning to repeat something verbatim.
Quines are used as a test of a programming language’s ability to handle self-referential code. In Python, a Quine is relatively easy to write.
All you should know is to surround your code with quotes and then use the print statement to output the code.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn