How to Solve the Issue - Python Program Closes Immediately
- Common Reasons for Immediate Closure
- Method 1: Using Input to Pause Execution
- Method 2: Running Python Scripts in Command Prompt or Terminal
- Method 3: Adding Exception Handling
- Conclusion
- FAQ
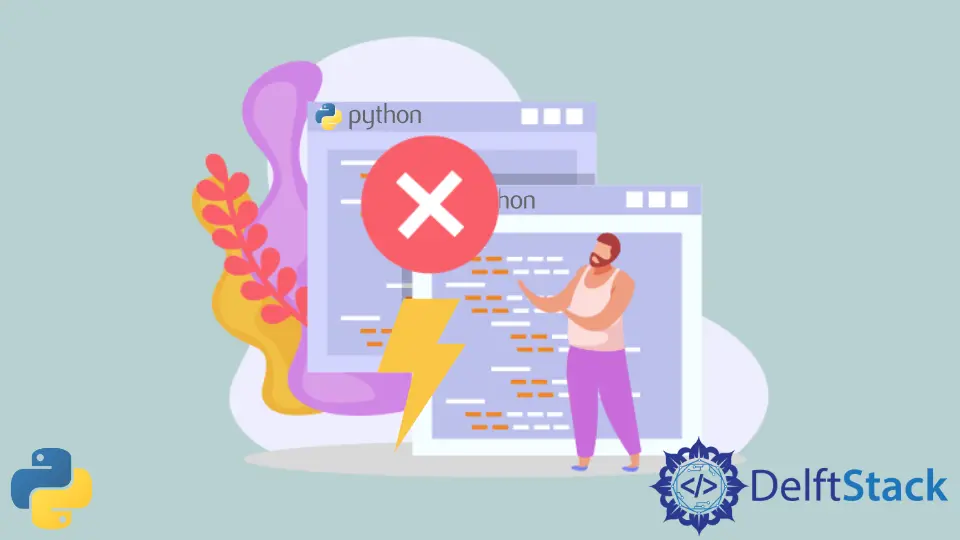
When you’re developing a Python application, you might encounter a frustrating issue: your program closes immediately after running. This sudden termination can leave you puzzled, especially if your code seems correct. Understanding why this happens is crucial for debugging and improving your coding skills.
In this tutorial, we will explore several methods to address the issue of a Python program closing immediately. We’ll cover straightforward solutions, including how to pause the program execution so you can see any error messages or outputs before the window closes. By the end of this article, you’ll have a better grasp of how to troubleshoot this common problem effectively.
Common Reasons for Immediate Closure
Before diving into solutions, let’s briefly discuss why a Python program might close immediately. Often, this occurs when the script encounters an error, or if it runs without any input prompts, causing it to execute and terminate quickly. If you’re running your Python script from an IDE, you may not notice the closure, but when executed from a command line, the window may close before you can read the output.
Method 1: Using Input to Pause Execution
One of the simplest methods to prevent your Python program from closing immediately is by using the input()
function. This function waits for user input, which effectively pauses the program. Here’s a basic example:
print("Hello, World!")
input("Press Enter to exit...")
Output:
Hello, World!
Press Enter to exit...
In this example, the program prints “Hello, World!” and then waits for the user to press Enter. This simple addition allows you to see the output before the program terminates. It’s particularly useful when you’re running your script in a command-line interface where the window might close immediately after execution.
Using input()
can also help you debug by allowing you to see any error messages that may appear before the program closes. If there’s an error in your code, you can fix it before running the program again. This method is easy to implement and can save you from a lot of confusion.
Method 2: Running Python Scripts in Command Prompt or Terminal
Another effective way to manage the immediate closure of your Python program is to run your script directly in a command prompt or terminal. This way, the terminal window remains open after the script finishes executing. Here’s how you can do it:
- Open your command prompt (Windows) or terminal (Mac/Linux).
- Navigate to the directory where your Python script is located using the
cd
command. - Run your script by typing
python your_script.py
.
Output:
Hello, World!
When you execute the command as shown, the terminal will display the output of your script and remain open until you manually close it. This approach is particularly beneficial for longer scripts or for debugging, as it allows you to review any messages or errors that occur during execution.
By running your script this way, you can easily see what’s happening and make adjustments as necessary. It’s a straightforward method that can significantly enhance your debugging experience.
Method 3: Adding Exception Handling
If your Python program closes immediately due to an unhandled exception, you can implement exception handling to capture and display error messages. This method helps you identify the exact cause of the issue. Here’s a simple example:
try:
print("Welcome to the program!")
x = 1 / 0 # This will raise a ZeroDivisionError
except Exception as e:
print(f"An error occurred: {e}")
finally:
input("Press Enter to exit...")
Output:
Welcome to the program!
An error occurred: division by zero
Press Enter to exit...
In this code, the try
block contains the main logic of the program. If an error occurs (like dividing by zero), the except
block catches the exception and prints a friendly error message. The finally
block ensures that the program waits for user input before closing, allowing you to read the error message.
This method not only prevents your program from closing immediately but also provides valuable feedback on what went wrong. By incorporating exception handling, you can improve your code’s robustness and make debugging much easier.
Conclusion
Experiencing your Python program closing immediately can be a frustrating hurdle, but with the right techniques, you can easily manage this issue. From using the input()
function to running scripts in a terminal, and implementing exception handling, there are multiple ways to keep your program open long enough for you to see the output or any error messages. By applying these methods, you can enhance your debugging skills and improve your overall programming experience.
FAQ
-
Why does my Python program close immediately?
Your Python program may close immediately due to an unhandled error or because it finishes executing without any prompts for user input. -
How can I see error messages in my Python script?
You can use theinput()
function to pause execution, or implement exception handling to catch and display error messages. -
Is it better to run Python scripts from an IDE or the command line?
Running scripts from the command line can help you see output and error messages more clearly, as the terminal will remain open after execution.
-
What is exception handling in Python?
Exception handling is a way to manage errors in your code usingtry
,except
, andfinally
blocks, allowing your program to respond gracefully to unexpected situations. -
Can I pause my program in other ways besides using input?
Yes, you can also implement time delays using thetime.sleep(seconds)
function, which pauses execution for a specified number of seconds.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn