How to Print Stack Trace in Python
-
Print Stack Trace in Python Using
traceback
Module -
Print Stack Trace in Python Using the
logging.exception()
Method
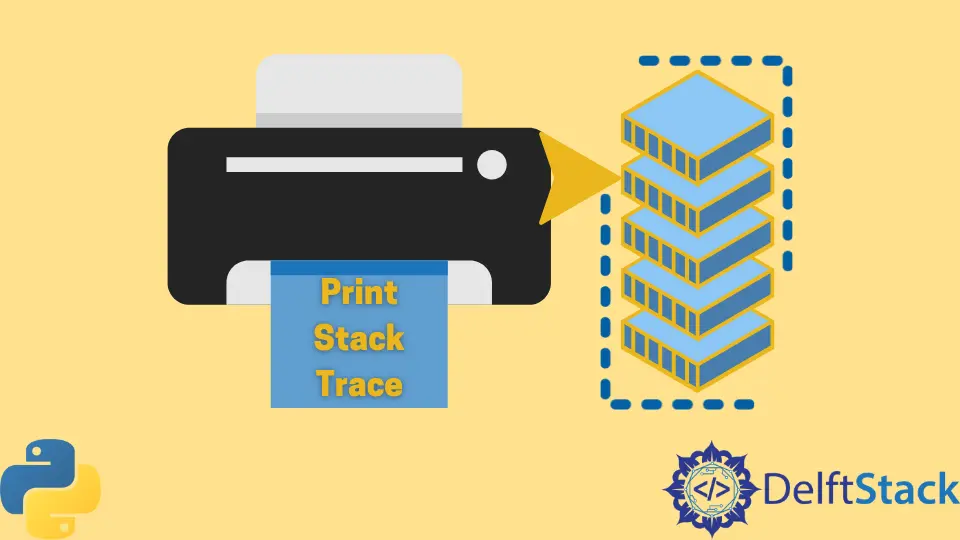
In this tutorial, we will look into various methods to print the stack trace without stopping the execution of the program in Python.
A stack trace contains a list of active method calls at a specific time. We can print the stack trace in Python using the following methods.
Print Stack Trace in Python Using traceback
Module
The traceback
module provides the functionalities to extract, format, and print stack traces in Python. The traceback.format_exc()
method returns a string that contains the information about exception and stack trace entries from the traceback object.
We can use the format_exc()
method to print the stack trace with the try
and except
statements. The example code below demonstrates how to print the stack trace using the traceback.format_exc()
method in Python.
import traceback
import sys
try:
myfunction()
except Exception:
print(traceback.format_exc())
Output:
Traceback (most recent call last):
File "C:\Test\test.py", line 5, in <module>
myfunction()
NameError: name 'myfunction' is not defined
Instead of using the print()
function, we can also use the logger.debug()
method to log the output, as logging can make debugging easier. We can log the stack trace in Python by using the logger.debug()
method in the following method.
import logging
import traceback
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
try:
myfunction()
except Exception:
logger.debug(traceback.format_exc())
Output:
DEBUG:__main__:Traceback (most recent call last):
File "C:\Test\test.py", line 8, in <module>
myfunction()
NameError: name 'myfunction' is not defined
Print Stack Trace in Python Using the logging.exception()
Method
We can also use the logging.exception()
method of the logging
module to get the stack trace in Python. The logging.exception()
method logs the message containing the exception information. We can use it to print stack trace in Python in the following way.
import logging
import traceback
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger(__name__)
try:
myfunction()
except Exception:
logging.info("General exception noted.", exc_info=True)
Output:
INFO:root:General exception noted.
Traceback (most recent call last):
File "C:\Test\test.py", line 8, in <module>
myfunction()
NameError: name 'myfunction' is not defined