How to Read a Text File and Print Its Contents in Python
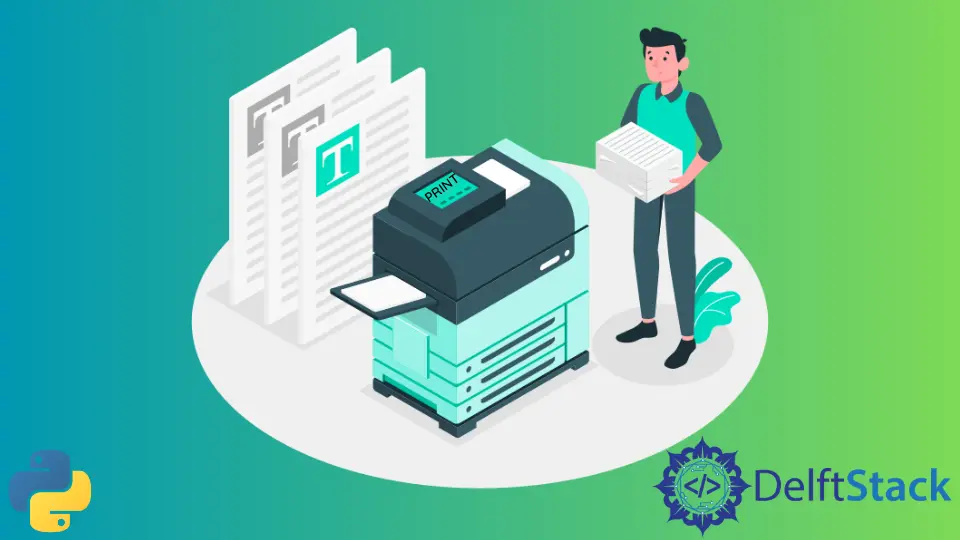
This article will demonstrate how to read a text file and print its contents to the screen using Python.
Cautions About Reading a File in Python
Do Not Open a Binary File
The method described in this article applies only to text files. Python modifies the end of line characters when reading a file; therefore, binary files must never be opened like this.
Read the official Python documentation on Reading and Writing Files for more details.
Avoid Reading the Whole File at Once
It is better to read a text file one line at a time and print each line to the screen before reading the next line. This will ensure that even files larger than memory are completely printed to the screen.
Read a Text File and Print Its Contents in Python
Create a File Object
The first step is to create a file object in read-only mode using the open()
function. The first argument is the file name and path; the second argument is the mode.
The function has other arguments, the most common of which is encoding
. If omitted, the default is platform-dependent.
It is common to use encoding = 'utf-8'
.
Example Code:
# Create the file object.
# Give the correct filename with path in the following line.
file_object = open("path_to_TEXT_file.txt", "r", encoding="utf-8")
Loop Over and Print the Strings in the File Object
Python provides a very efficient way to read and print each line of a file object.
A simple for
loop is used for this purpose. The word string
in the following code is just a variable name; the code loops over all the lines in the file.
Example Code:
# Loop over and print each line in the file object.
for string in file_object:
print(string)
Explanation:
- The loop prints one line of the text file on each iteration.
- Each line ends where the new line character is encountered.
- The loop terminates when all lines have been printed.
Close the File Object
After the code prints all the lines in the file, the object must be closed to free up memory.
Example Code:
# Close the file object.
file_object.close()
Complete Example Code:
# python3
# coding: utf-8
# Create the file object.
# Give the correct filename with path in the following line.
file_object = open("path_to_TEXT_file.txt", "r", encoding="utf-8")
# Loop over and print each line in the file object.
for string in file_object:
print(string)
# Close the file object.
file_object.close()