How to Print With Column Alignment in Python
-
Using
%
Formatting for Column Alignment -
Using
format()
for Column Alignment - Using f-strings for Column Alignment
-
Using
expandtabs()
for Column Alignment -
Using
ljust()
for Left Alignment -
Using
tabulate
for Column Alignment -
Using
pandas
for Column Alignment - Conclusion
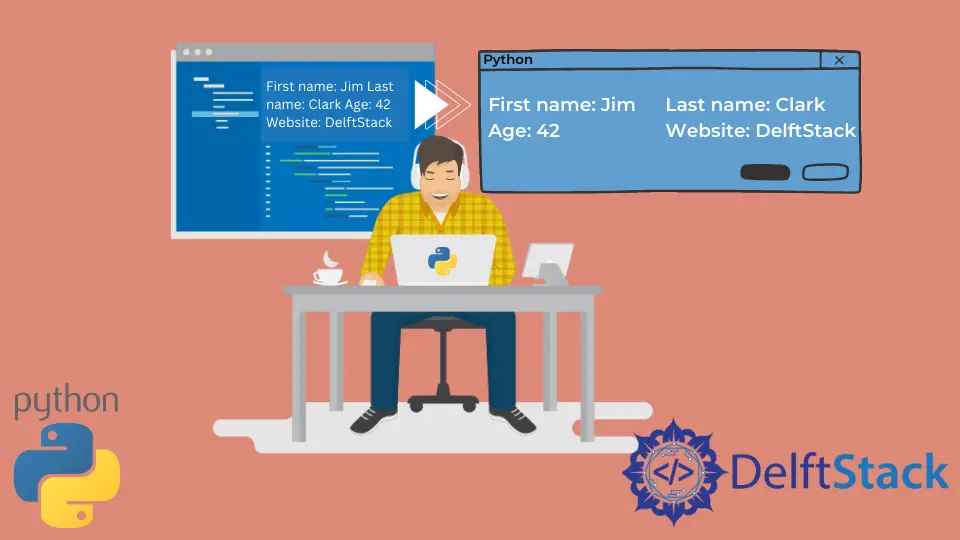
The print()
function has evolved in Python, transitioning from a statement in Python 2 to a function in Python 3. Formatting output correctly ensures clarity, especially when dealing with tabular data.
This tutorial explores various methods to print with column alignment in Python. If you need to print multiple arguments efficiently while aligning columns, refer to our guide on Printing Multiple Arguments in Python.
Using %
Formatting for Column Alignment
The %
formatting method is one of the oldest ways to format strings. Using %-*s
, we can define spacing that acts as the column width. The spacing must be manually adjusted for each row.
Example:
print("First Name: %-*s Last Name: %s" % (13, "Jim", "Clark"))
print("Age: %-*s Website: %s" % (20, "42", "DelftStack.com"))
Output:
First Name: Jim Last Name: Clark
Age: 42 Website: DelftStack.com
This approach provides basic alignment but requires careful spacing adjustments. The %
operator allows dynamic column width by using %-*s
, where *
represents a width determined at runtime. However, it requires manual spacing for each value, making it less flexible than newer formatting techniques.
Using format()
for Column Alignment
The format()
function provides a more flexible way to align text within columns. By defining fixed-width spaces within the curly braces, we can ensure consistent column alignment.
Example:
print("{:<10} {:<5} {:<15}".format("First Name:", "Jim", "Last Name: Clark"))
print("{:<10} {:<5} {:<15}".format("Age:", "42", "Website: DelftStack.com"))
Output:
First Name: Jim Last Name: Clark
Age: 42 Website: DelftStack.com
Using {:<10} {:<5} {:<15}
, we specify fixed-width fields that align text in a structured way. This method ensures that text is properly formatted regardless of its length.
If you’re working with numerical formatting alongside column alignment, you may find our guide on Formatting Numbers with Commas in Python useful.
Using f-strings for Column Alignment
Introduced in Python 3.6, f-strings offer a concise and readable way to format text while supporting alignment specifications.
Example:
print(f"{'First Name: ' + 'Jim':<25} Last Name: {'Clark'}")
print(f"{'Age: ' + '42':<25} Website: {'DelftStack.com'}")
Output:
First Name: Jim Last Name: Clark
Age: 42 Website: DelftStack.com
F-strings provide an intuitive approach to formatting text while reducing verbosity. This method supports alignment just like format()
, allowing :<
, :>
, and :^
for left, right, and center alignment, respectively. Since f-strings are evaluated at runtime, they are both efficient and easy to read, making them an excellent choice for column alignment.
Using expandtabs()
for Column Alignment
Tabs (\t
) can be used to structure output, and expandtabs()
allows explicit tab width control to adjust spacing dynamically.
Example:
print(("First Name: Jim" + "\t" + "Last Name: Clark").expandtabs(13))
print(("Age: 42" + "\t" + "Website: DelftStack.com").expandtabs(26))
Output:
First Name: Jim Last Name: Clark
Age: 42 Website: DelftStack.com
This method is useful when working with tab-separated values (TSV
), but since tab width depends on terminal settings, expandtabs()
may not always produce consistent results across different environments.
Using ljust()
for Left Alignment
Python provides ljust()
, rjust()
, and center()
methods for text alignment. The ljust()
method ensures left alignment within a fixed width. For a deeper understanding of string padding techniques, check out Padding Strings with Spaces in Python.
Example:
print("First Name: Jim".ljust(40) + "Last Name: Clark")
print("Age: 42".ljust(40) + "Website: DelftStack.com")
Output:
First Name: Jim Last Name: Clark
Age: 42 Website: DelftStack.com
Using ljust(40)
, each string is padded with spaces to ensure a consistent column width.
Using tabulate
for Column Alignment
The tabulate
module provides a simple way to format structured data into aligned columns. It is especially useful for tabular data that requires consistent spacing without manually adjusting alignment for each row.
To use tabulate
, you need to install it first. You can install the module using pip:
pip install tabulate
Once installed, tabulate
automatically determines the appropriate column width and formats the output neatly. By using tablefmt="plain"
, we ensure that the output remains simple and readable in text-based environments without adding unnecessary table borders.
Tabulate automatically determines the appropriate column width and formats the output neatly. By using tablefmt="plain"
, we ensure that the output remains simple and readable in text-based environments without adding unnecessary table borders.
Example:
from tabulate import tabulate
data = [["First Name:", "Jim", "Last Name: Clark"],
["Age:", "42", "Website: DelftStack.com"]]
print(tabulate(data, tablefmt="plain"))
Output:
First Name: Jim Last Name: Clark
Age: 42 Website: DelftStack.com
Using pandas
for Column Alignment
When dealing with large datasets, the pandas library provides a structured way to format and align tabular data efficiently. If you’re working with structured data, you may also need to Convert a List to a Pandas DataFrame for efficient processing. Unlike manual formatting, pandas ensures consistent column alignment regardless of the dataset size.
Example:
import pandas as pd
# Create a DataFrame with fixed column widths for better alignment
data = {"Column 1": ["First Name:", "Age:"],
"Column 2": ["Jim", "42"],
"Column 3": ["Last Name: Clark", "Website: DelftStack.com"]}
df = pd.DataFrame(data)
# Adjust column width for better alignment
col_width = max(df.applymap(lambda x: len(str(x))).max()) + 2
df_aligned = df.applymap(lambda x: str(x).ljust(col_width))
print(df_aligned.to_string(index=False, header=False))
Calculating the Maximum Column Width
col_width = max(df.applymap(lambda x: len(str(x))).max()) + 2
- This step ensures uniform spacing across columns by determining the longest string length in the dataset.
- The
applymap()
function applieslen(str(x))
to every value in the DataFrame, ensuring string lengths are calculated correctly. - The
max()
function extracts the largest value, and+2
adds extra padding for readability.
Applying Left Alignment to Each Cell
df_aligned = df.applymap(lambda x: str(x).ljust(col_width))
- The
applymap()
function is again used, this time applyingljust(col_width)
to each value. ljust(col_width)
ensures all values are left-aligned within a uniform column width, preventing misalignment.
Printing the Table as a Plain Text Output
print(df_aligned.to_string(index=False, header=False))
to_string(index=False, header=False)
removes the DataFrame’s index and headers to create a clean, column-aligned output.
Conclusion
There are multiple ways to achieve column alignment in Python, each suited to different use cases. Below is a comparison of the methods discussed:
Method | Flexibility | Readability | Best Use Case |
---|---|---|---|
% Formatting |
Low | Moderate | Simple cases with fixed widths |
format() |
High | High | General-purpose formatting |
f-strings | High | Very High | Readable and efficient formatting |
expandtabs() |
Moderate | Low | Tab-based alignment |
ljust() |
Moderate | High | Ensuring left alignment |
tabulate |
High | Very High | Formatting structured text tables |
pandas |
High | High | Handling large datasets |
Each method has its own advantages:
format()
and f-strings offer flexibility and readability, making them ideal for general-purpose text formatting.expandtabs()
can be useful for aligning tab-separated values, but alignment may vary based on terminal settings.ljust()
provides a simple way to left-align text when formatting requirements are minimal.tabulate
is excellent for generating structured tables with minimal effort.pandas
is best suited for large datasets where structured alignment is required without manual adjustments.
For basic text formatting, format()
or f-strings are the best options. For structured tabular data, tabulate
and pandas
provide automated formatting that ensures consistent alignment. If you’re working with matrices, check out Printing Matrices in Python for more structured output techniques. Choosing the right method depends on the complexity of the data and the output requirements.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn