How to Pass by Reference in Python
- The Definition of the Pass by Reference in Python Function
- Pass by Reference Example in Python
- Explanation
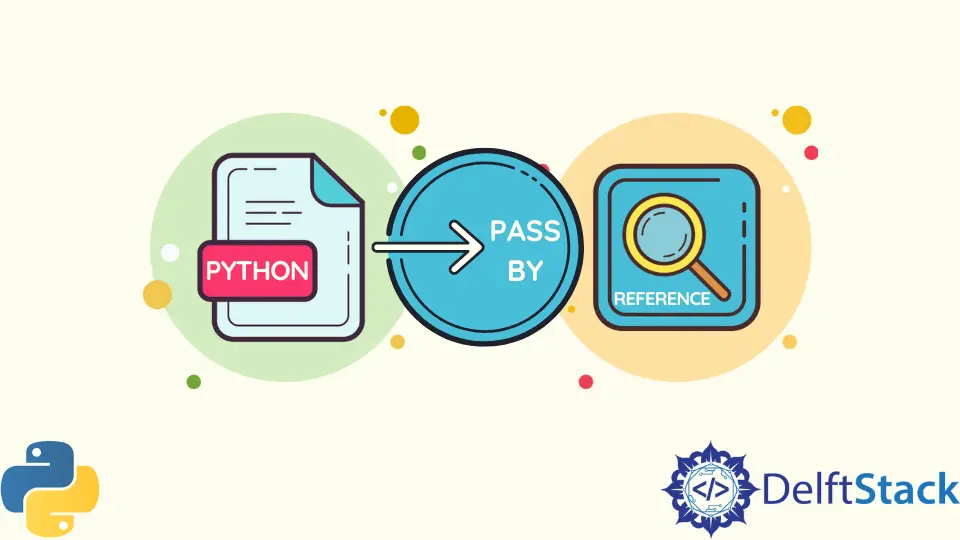
In this guide, we will demonstrate what you need to know about pass by reference. We’ve included a sample program below, which you can follow to understand this function better.
The Definition of the Pass by Reference in Python Function
There are many ways to define an argument in a function in Python; one of these processes is the pass by reference. The word Pass
here means to pass or give an argument to a function. Then by reference
means that the argument passed to a function is basically referred to as an existing variable instead of a separate copy of that variable. In this method of defining an argument in a function, the variable that has been referred to is affected mostly by any operation performed.
Pass by Reference Example in Python
def fun(x):
x.append("Sam")
print("While calling the function:", x)
x = ["Hello"]
print("Before calling the function:", x)
fun(x)
print("After calling the function:", x)
Output:
Before calling the function: ['Hello']
While calling the function: ['Hello', 'Sam']
After calling the function: ['Hello', 'Sam']
Explanation
In the above example, a function is first defined with a variable x
. Here, the append
method is used with x
to add to an element name sam
. After that, a list is made using the element x
in which there is only one element, i.e., hello
. Upon printing the list, the function that was initially defined is called along with its argument x
. After calling the function, note that the appended element in the function itself has been added to the list x
.
This process depicts how pass by reference
works. The function always affects the mutable objects (objects that can change their value or state) stored in a variable used as the function argument.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn