How to Use the pass Statement in Python
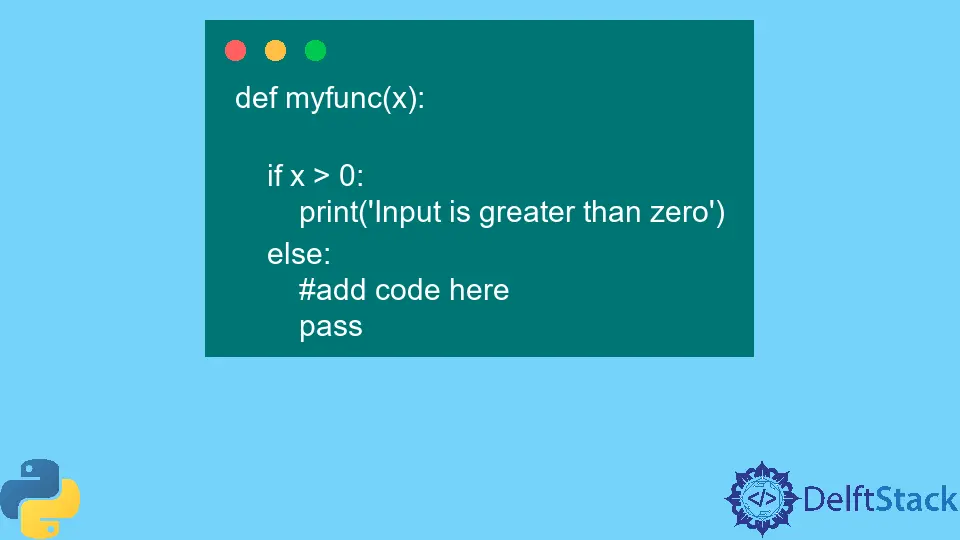
This tutorial will explain the details and use of the pass
statement in Python. In almost every high-level programming language, various statements are used for different purposes. Programming languages like C, C++, JAVA, C#, and Python use statements like if ... else
, return
, break
statement, etc., and all these statements are used for different purposes.
However, the pass
statement is a Python-specific statement used as a placeholder when the user wants the program to do nothing.
Use the pass
Statement in Python
The pass
statement is used in Python when some code is required syntactically, but the user does not want the program to do anything. There can be different reasons behind it; one can be that the user plans to add the code later, or it can be used to ignore some exceptions raised during the run time.
The below example code demonstrates how to use the pass
statement in case where user wants to add the code later:
def myfunc(x):
if x > 0:
print("Input is greater than zero")
else:
# add code here
pass
If the pass
statement was not added in the above code example, the compiler would have returned SyntaxError
or IdentationError
.
We can also use the pass
statement to ignore an exception in Python as demonstrated in the example code provided below:
def divide(x, y):
z = None
try:
z = x / y
except:
pass
return z
divide(6, 0)
In the above example code, we have used the pass
statement along try
and except
statements to handle the ZeroDivisionError
and prevent the code from crashing.