How to Calculate Partial Derivatives in Python Using Sympy
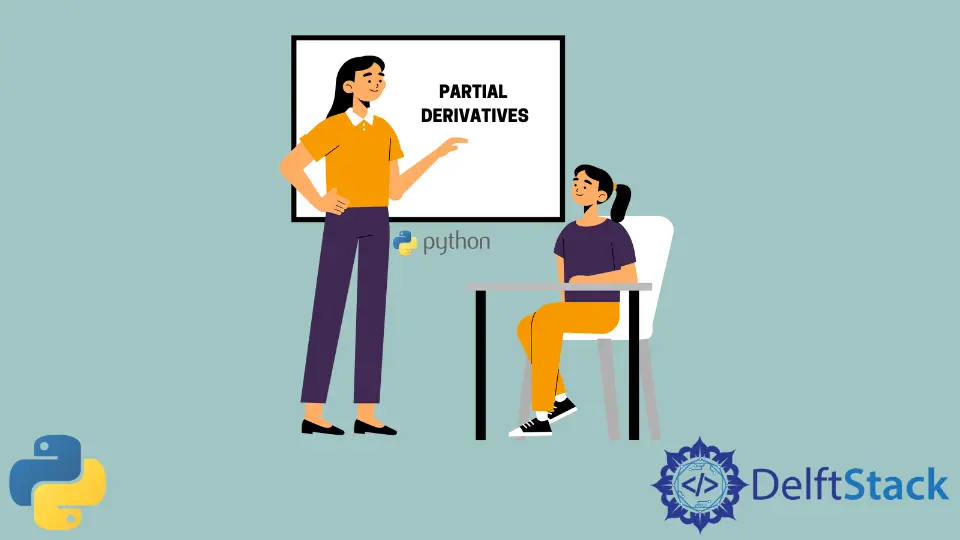
A partial derivative is a function’s derivative that has two or more other variables instead of one variable. Because the function is dependant on several variables, the derivative converts into the partial derivative.
For example, where a function f(b,c)
exists, the function depends on the two variables, b
and c
, where both of these variables are independent of each other. The function, however, is partially dependant on both b
and c
. Therefore, to calculate the derivative of f
, this derivative will be referred to as the partial derivative
. If you differentiate the f function with reference to b, you will use c as the constant. Otherwise, if you differentiate f regarding c, you will take b as the constant instead.
In Python, the Sympy
module is used to calculate the partial derivative in a mathematical function. This module uses symbols to perform all different kinds of computations. It can also be used to solve equations, simplify expressions, compute derivatives and limits, and other computations.
Sympy
needs to be manually installed before it can be used. Therefore, cd to your computer terminal and run the following command to install the sympy
package.
pip install sympy
To use
sympy
to compute a partial derivative, you first need to import thesympy
package from symbols.
The computer evaluates the computation of values differently from how they are put down on a piece of paper. Therefore, symbols here will be in the form of variables that hold the real values to be evaluated. Thus, during the computation, the computer manipulates the variable to the value it is attached to.
Now, let’s use the following example to derive the partial derivative of the function.
f(a, b, c) = 5ab - acos(c)+ a^2 + c^8b
part_deriv(function = f, variable = a)
The expected output after differentiating the function to its partial derivative is 2*a + 5*b - cos(c)
.
To evaluate the partial derivative of the function above, we differentiate this function in respect to a
while b
and c
will be the constants.
from sympy import symbols, cos, diff
a, b, c = symbols("a b c", real=True)
f = 5 * a * b - a * cos(c) + a ** 2 + c ** 8 * b
# differntiating function f in respect to a
print(diff(f, a))
Output:
2*a + 5*b - cos(c)