How to Fix Operands Could Not Be Broadcast Together With Shapes Error in Python
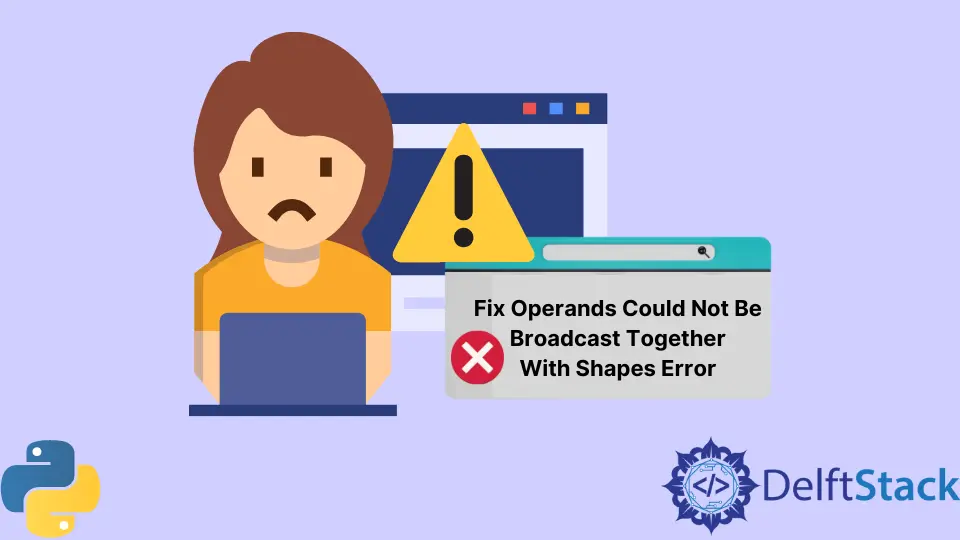
In Python, numpy arrays with different shapes cannot be broadcast together. It means you cannot add two 2D arrays with different rows and columns.
But there is a way through which you can do that. Take a look.
Fix operands could not be broadcast together with shapes
Error in Python
You can not add or multiply two 2D arrays with different shapes. Take a look at the following code.
import numpy as np
# Addition Example
# 2d array with shape 2 rows and 3 columns
array_1 = np.array([[1, 1, 1], [1, 1, 1]])
# 2d array with shape 3 rows and 2 columns
array_2 = np.array([[1, 1], [1, 1], [1, 1]])
# Addition applying on the arrays
array_Addition = array_1 + array_2
As you can see, there are two 2D arrays with different shapes.
The first array has two rows and three columns, and the second array is three rows and two columns. Adding them will give this error operands could not be broadcast together with shapes
.
It works the same as adding two matrices in mathematics.
The first element of the first array is added with the first element of the second array, and the second element will be added with the second one. So, if the shapes aren’t matched, it will give an error.
Therefore, we need to use the reshaping functionalities to have the same shapes for the two 2D arrays. In the following code, we are reshaping the second array according to the shapes of the first.
Once the shapes are the same, you can add the two arrays.
import numpy as np
# Addition Example
# 2d array with shape 2 rows and 3 columns
array_1 = np.array([[1, 1, 1], [1, 1, 1]])
# 2d array with shape 3 rows and 2 columns
array_2 = np.array([[1, 1], [1, 1], [1, 1]])
# changing the shape of array_2 according to the Array_1 or Array_1 according to Array_2
array_2 = array_2.reshape((2, 3))
# again Addition applying on the arrays
array_Addition = array_1 + array_2
print(array_Addition)
Output:
[[2 2 2]
[2 2 2]]
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python