How to Import OpenSSL in Python
Preet Sanghavi
Feb 02, 2024
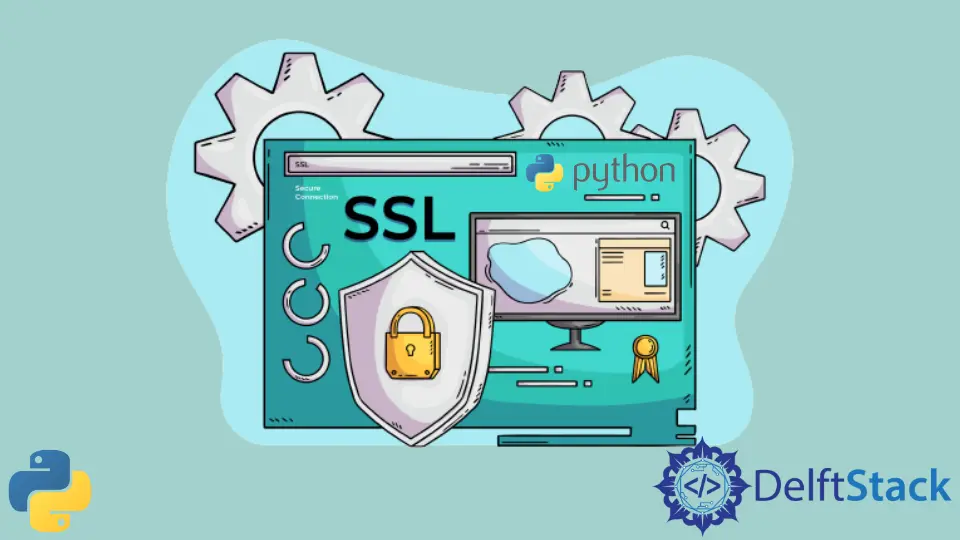
In this tutorial, we aim at learning how to use OpenSSL in Python.
Install OpenSSL Python Library
We need to install the OpenSSL library to get started. We do this by running the following command in cmd.
pip install pyopenssl
Import OpenSSL in Python
After installing the above package, we need to import the SSL
function from the OpenSSL library.
from OpenSSL import SSL
The above code will import the SSL library, which we can use to perform various SSL certificate-related operations.
We will now print the certificate path location using the SSL library to verify that the pyOpenSSL
package was successfully installed and the library was successfully imported.
print(SSL._CERTIFICATE_PATH_LOCATIONS)
We get the below output on running the above command.
['/etc/ssl/certs']
Thus we can successfully work with SSL certificates using the above method.
Author: Preet Sanghavi