How to Fix Object Is Not Subscriptable Error in Python
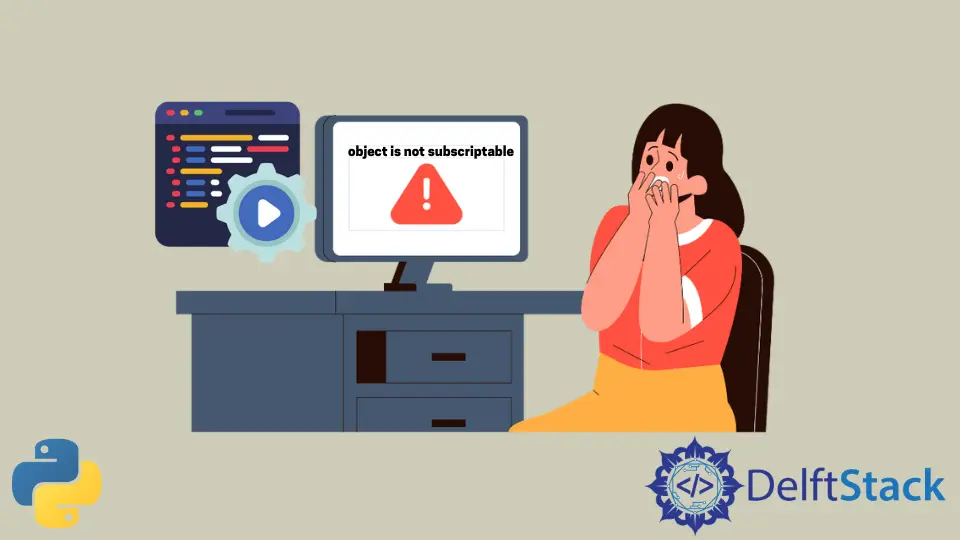
In Python, the object is not subscriptable
error is self-explanatory. If you came across this error in Python and looking for a solution, keep reading.
Fix the object is not subscriptable
Error in Python
First, we need to understand the meaning of this error, and we have to know what is meant by subscriptable.
A subscript is a symbol or number in a programming language to identify elements. So, by object is not subscriptable
, it is obvious that the data structure does not have this functionality.
For instance, take a look at the following code.
# An integer
Number = 123
Number[1] # trying to get its element on its first subscript
Running the code above will result in an error since an integer does not have multiple values. Therefore, a need for subscript in integer does not make sense. Let’s see some more examples.
# Set always has unique Elements
Set = {1, 2, 3}
# getting second index of set #wrong
Set[2]
We initialized a set with some values; don’t mistake it for a list or an array. A set does not have subscripts. Meaning, the above code will also give the same error.
We can not display a single value from a set. If we use a loop to print the set values, you will notice it does not follow any order.
There is no index identifying its value. The output of the following code will give different order output.
# Set always has unique Elements
Set = {1, 2, 4, 5, 38, 9, 88, 6, 10, 13, 12, 15, 11}
# getting second index of set
for i in Set:
print(i)
When it comes to string or list, you can use subscript to identify each element. That is like printing and getting a value from a simple array. Take a look.
# string variable
string = "Hello I am Python"
print(string[4])
Output:
o
The above code will run successfully, and the output will be o
as it is present on the string’s fifth index/subscript (0-4). This object is subscriptable.
# function which returns a list
def my_Func():
return list(range(0, 10))
# correct
print(my_Func()[3])
Output:
3
In the code above, we have a function that returns a list that is also subscriptable. As you can see, we are displaying the third element of the list and using the subscript and index method.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python