How to Get a Negation of a Boolean in Python
-
Use the
not
Operator to Negate a Boolean in Python -
Use the
operator.not_()
Function From theoperator
Module to Negate a Boolean in Python -
Use the
~
Operator to Negate Boolean Values of a NumPy Array in Python -
Use the
bitwise_not()
Function From the NumPy Library to Negate a Boolean Value -
Use the
invert()
Function From the NumPy Library to Negate a Boolean Value in Python -
Use the
logical_not()
Function From the NumPy Library to Negate a Boolean Value in Python
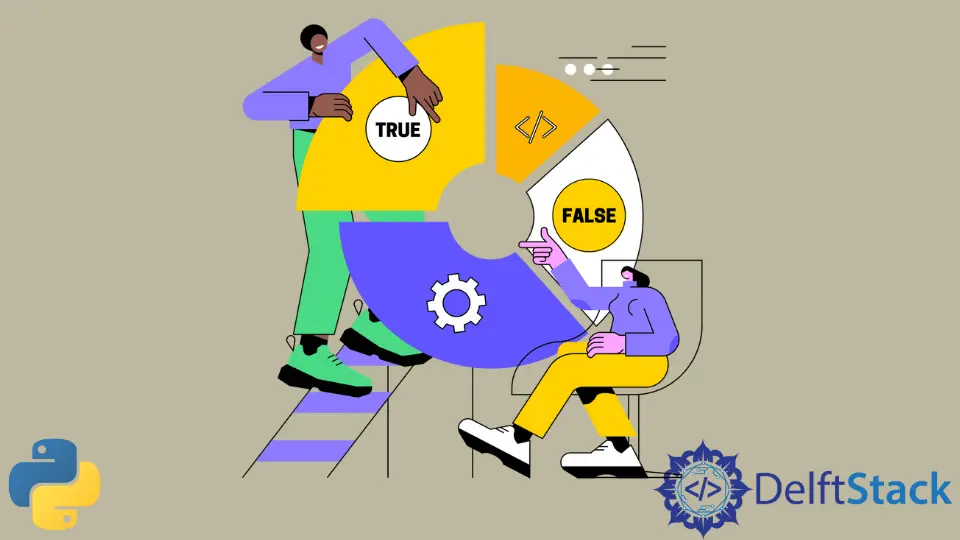
There are various types of built-in data types in Python; one is the boolean
data type. The boolean
data type is a built-in data type used to define true and false values of the expressions with the keywords True
and False
.
There are many cases while dealing with boolean operators or boolean sets/arrays in Python, where there is a need to negate the boolean value and get the opposite of the boolean value.
This tutorial will demonstrate different ways to negate a Boolean value in Python.
Use the not
Operator to Negate a Boolean in Python
The not
operator in Python helps return the negative or the opposite value of a given boolean value. This operator is used by placing the not
operator as a prefix of a given boolean expression. Check the example below.
a = 1
print(bool(a))
print(not a)
Output:
True
False
Here, the bool()
function is used. It returns the boolean value, True
or False
, of a given variable in Python. The boolean values of the numbers 0
and 1
are set to False
and True
as default in Python.
So, using the not
operator on 1
returns False
, i.e., 0
. Also, note that the not
operator can be used in the print
statement itself.
Use the operator.not_()
Function From the operator
Module to Negate a Boolean in Python
The operator
module in Python is used to provide various functions that are related to intrinsic operators of Python.
The operator.not_()
function takes a boolean value as its argument and returns the opposite of that value. Take a look at the example here.
import operator
print(operator.not_(True))
Output:
False
This function is also used to negate booleans values stored in a list or an array.
import operator
bool_values = [True, True, False, True, False]
negate_bool = map(operator.not_, bool_values)
print(list(negate_bool))
Output:
[False, False, True, False, True]
In the example above, the map()
function is also used. This process is used to perform an operation or apply a function to all the items of a defined iterator, such as a list, a tuple, or a dictionary.
Use the ~
Operator to Negate Boolean Values of a NumPy Array in Python
A NumPy array is a list of values of the same type with pre-defined index values. The shape of the NumPy array is defined by a tuple of integers that gives the size of the array.
The ~
operator is also called the tilde operator. This operator is the bitwise negation operator that takes a number as a binary number and converts all the bits to their opposite values.
For example, 0
to 1
and 1
to 0
. In Python, 1
denotes True
, and 0
denotes False
. So, the tilde operator converts True
to False
and vice-versa. Here’s an example to demonstrate this process.
import numpy as np
b = np.array([True, True, False, True, False])
b = ~b
print(b)
Output:
[False False True False True]
Use the bitwise_not()
Function From the NumPy Library to Negate a Boolean Value
The bitwise_not()
function helps in assigning a bitwise NOT
operation to an element or an array of elements.
import numpy as np
b = np.array([True, True, False, True, False])
b = np.bitwise_not(b)
print(b)
Output:
[False False True False True]
Here, a NumPy array is used, but a single boolean value can also be stored in the input variable.
Use the invert()
Function From the NumPy Library to Negate a Boolean Value in Python
The invert()
function helps in the bitwise inversion of an element or an array of elements. This function also returns the bitwise NOT
operation.
Example:
import numpy as np
b = np.array([True, True, False, True, False])
b = np.invert(b)
print(b)
Output:
[False False True False True]
Use the logical_not()
Function From the NumPy Library to Negate a Boolean Value in Python
The logical_not()
function of the NumPy library basically returns the True
value of the NOT
value of an element or an array of elements(element-wise).
Example:
import numpy as np
b = np.array([True, True, False, True, False])
b = np.logical_not(b)
print(b)
Output:
[False False True False True]
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn