Python MRO (Method Resolution Order)
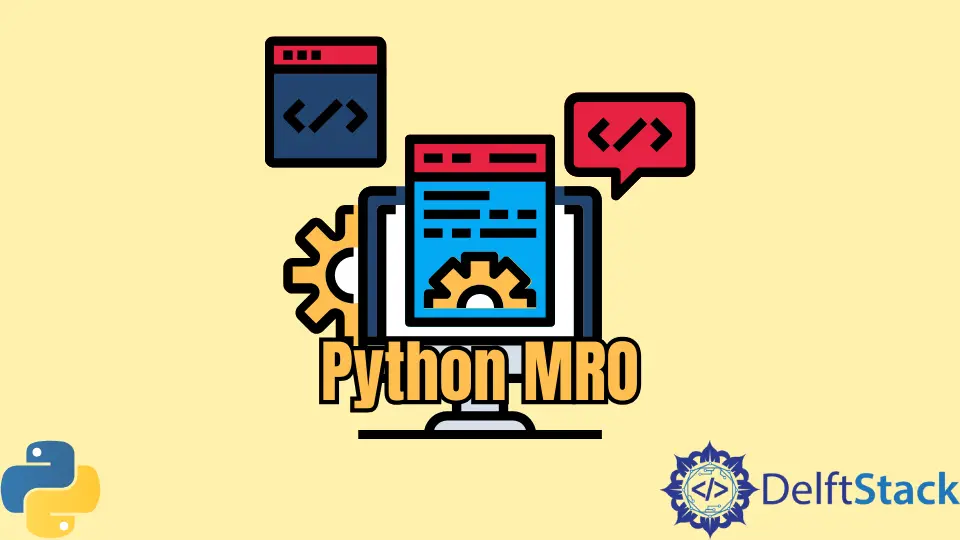
Multiple inheritances mean that a single subclass can inherit more than one class, and the subclass will be authorized to access the attributes and functions unless they aren’t private to that specific class. The MRO technique is used there to search the order of the classes being executed.
In this article, we will learn Python MRO(Method Resolution Order).
MRO (Method Resolution Order) in Python
MRO is the order in Python where a method is looked for in the class hierarchy. Mostly it is used to look for methods and attributes in the parent classes of a subclass.
First, the attribute or method is searched within the subclass, following the specified order while inheriting. This order is also known as the Linearization of a class, and a set of rules is applied while checking the order.
While inheriting from other classes, the compiler needs a proper way to resolve the methods called via an instance of the class. It plays an important role in multiple inheritances when we have the same function in more than one parent of base classes.
Code Example:
class A(object):
def dothis(self):
print("This is A class")
# Class B is inheriting Class A
class B(A):
def dothis(self):
print("This is B class")
# Class C is inheriting Class B
class C(B):
def dothis(self):
print("This is C class")
# Class D is inheriting Class C and B
class D(C, B):
def dothis(self):
print("This is D class")
# creating the object of Class D
d_obj = D()
# calling funtion dothis()
d_obj.dothis()
print(D.mro())
Output:
This is D class
[<class '__main__.D'>, <class '__main__.C'>, <class '__main__.B'>, <class '__main__.A'>, <class 'object'>]
In the above example, the function dothis()
is available in every class. When you call it using the object d_obj
of class D
, it first checks it in the current class.
If it is there, it will return the function. Otherwise, it will keep looking into the parent classes according to the rules of the mro()
function.
Furthermore, to know the order of classes, you call the mro()
function using the current class name as demonstrated in the above example, D.mro()
. The current order is Class D -> Class C -> Class B -> Class A
, and you can change it accordingly during inheritance.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn