How to Solve the ModuleNotFoundError: No Module Named 'cPickle' Error in Python
- Understanding the cPickle Module
- Solution: Updating Your Import Statements
- Solution: Checking Your Python Version
- Solution: Creating a Virtual Environment
- Conclusion
- FAQ
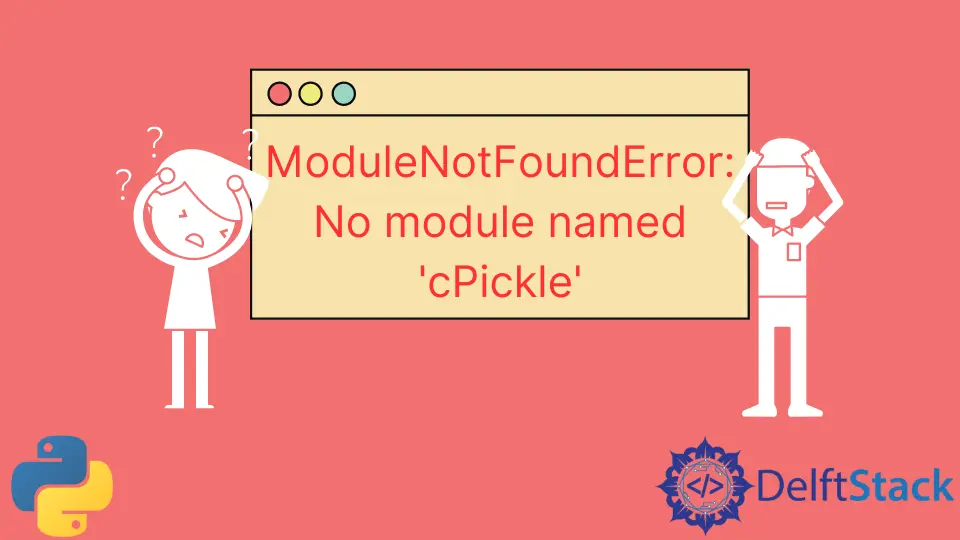
When working with Python, encountering errors can be frustrating, especially when they disrupt your workflow. One common error that developers face is the ModuleNotFoundError: No module named ‘cPickle’. This error typically arises when you’re trying to import the cPickle module, which is not available in Python 3. Instead, its functionality has been integrated into the standard pickle module. If you’re transitioning from Python 2 to Python 3, this can catch you off guard.
In this article, we’ll explore the causes of this error and provide you with effective solutions to resolve it, ensuring a smoother coding experience.
Understanding the cPickle Module
The cPickle module was part of Python 2, designed to serialize and deserialize Python objects efficiently. However, with the advent of Python 3, cPickle was merged into the pickle module, which means you need to adjust your imports accordingly. If you’re still trying to use cPickle in Python 3, you’ll encounter the ModuleNotFoundError. To resolve this, you simply need to replace cPickle with pickle in your import statements.
Solution: Updating Your Import Statements
The first step in resolving the ModuleNotFoundError is to update your import statements. Instead of importing cPickle, you should import the pickle module. This change is straightforward and can be done in just a few seconds.
Here’s how you can modify your code:
import pickle
data = {'key': 'value'}
serialized_data = pickle.dumps(data)
deserialized_data = pickle.loads(serialized_data)
print(deserialized_data)
Output:
{'key': 'value'}
By replacing import cPickle
with import pickle
, you ensure that your code is compatible with Python 3. The pickle.dumps()
function converts a Python object into a byte stream, while pickle.loads()
does the reverse, converting the byte stream back into a Python object. This simple change resolves the error and allows your code to run smoothly.
Solution: Checking Your Python Version
Another important step is to verify the version of Python you are using. If you’re working in an environment where multiple Python versions are installed, it’s possible that your script is being executed with Python 2 instead of Python 3. This can lead to the ModuleNotFoundError because cPickle exists only in Python 2.
To check your Python version, you can run the following command in your terminal or command prompt:
python --version
Output:
Python 3.x.x
If you find that you’re using Python 2.x.x, you may want to switch your environment to Python 3. You can do this by explicitly calling python3
instead of python
in your command line. This ensures that your scripts run in the correct version.
Solution: Creating a Virtual Environment
If you’re still encountering issues, consider creating a virtual environment. Virtual environments allow you to manage dependencies for different projects separately, which can prevent conflicts between packages and modules. Here’s how to create a virtual environment using Python 3:
python3 -m venv myenv
source myenv/bin/activate
Output:
(myenv)
After activating your virtual environment, you can install any necessary packages. This is particularly useful if you’re working on a project that requires specific dependencies. Make sure to install the latest version of Python and related packages in this environment.
To deactivate the virtual environment, simply run:
deactivate
Output:
(myenv) $
Using a virtual environment can help you avoid the ModuleNotFoundError by isolating your project’s dependencies. This way, you can ensure that you’re using the correct version of Python and the necessary modules without interference from other projects.
Conclusion
In summary, the ModuleNotFoundError: No module named ‘cPickle’ error is a common issue for those transitioning from Python 2 to Python 3. By updating your import statements, checking your Python version, and utilizing virtual environments, you can effectively resolve this error and enhance your coding experience. Remember, adapting to changes in programming languages is part of the journey, and with these solutions, you’ll be well-equipped to tackle similar issues in the future.
FAQ
-
What is cPickle used for?
cPickle is used for serializing and deserializing Python objects, but it is only available in Python 2. -
Why do I get ModuleNotFoundError for cPickle in Python 3?
In Python 3, cPickle has been merged into the pickle module, so you should useimport pickle
instead. -
How can I check my Python version?
You can check your Python version by running the commandpython --version
in your terminal. -
What is a virtual environment in Python?
A virtual environment is a self-contained directory that allows you to manage dependencies for Python projects separately. -
How do I create a virtual environment?
You can create a virtual environment using the commandpython3 -m venv myenv
, followed bysource myenv/bin/activate
to activate it.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHubRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python