How to Fix Missing Parentheses in Print Error in Python
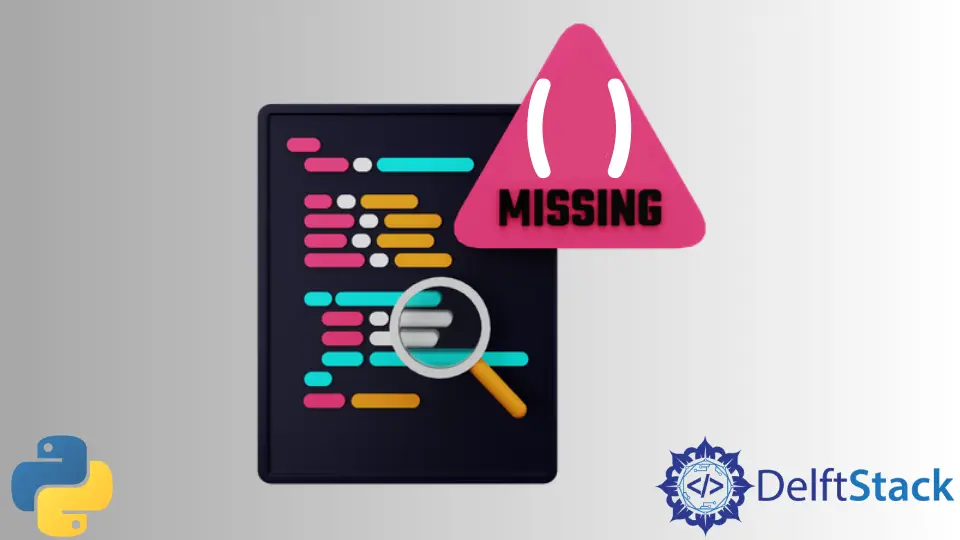
We will discuss the missing parentheses in call to 'print'
error in Python. This error is a compile-time syntax error.
See the code below.
print "Something"
Output:
SyntaxError: Missing parentheses in call to 'print'. Did you mean print("Something")?
Whenever this error is encountered, remember to use parentheses while printing.
For example,
print("Something")
Output:
Something
Let us now discuss what happened.
Python 3 was a major update for the Python language since a lot of new changes were introduced. One such change was the need to use the parentheses with the print()
function. In Python 2, there was no such need.
This change is because, in Python 2, the print
was a statement and was changed to a function in Python 3. That is why we need to use parentheses as we do in a normal function call.
This change was considered an improvement because it allowed adding parameters like sep
within the print()
function.
In earlier versions of Python 3, whenever the print()
function was encountered without parentheses, a generic SyntaxError: invalid syntax
error was raised. However, this was a little ambiguous because an invalid syntax error can be raised for many reasons.
The error was changed to SyntaxError: Missing parentheses in call to 'print'
to avoid any confusion.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Print
- How to Print Multiple Arguments in Python
- How to Print With Column Alignment in Python
- How to Print Subscripts to the Console Window in Python
- How to Print Quotes in Python
- How to Print % Sign in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python