Memory Profiler in Python
-
Monitor Memory Consumption Using
Memory Profiler
Module in Python -
Monitor Memory Consumption Using the
guppy
Module in Python
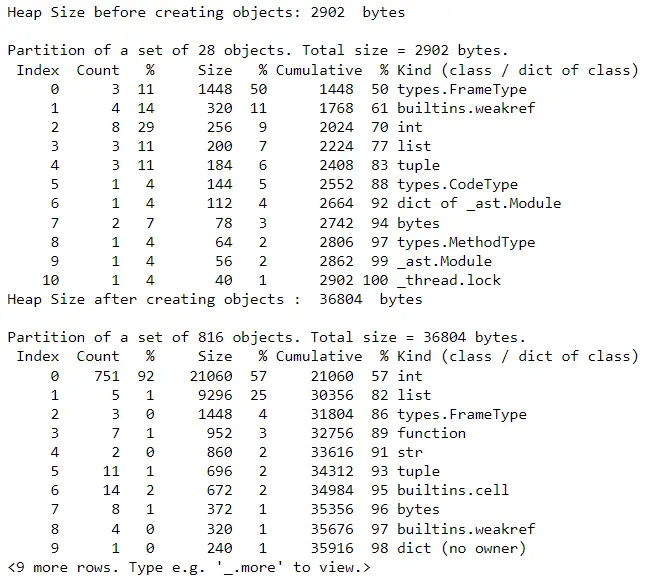
The Memory profilers
are the modules that monitor the memory consumption of code blocks.
When they are created, it shows the amount of memory taken by variables
, objects
, functions
, etc. This article will see Memory Profiler
and guppy
to analyze Python’s memory consumption.
Monitor Memory Consumption Using Memory Profiler
Module in Python
The Memory Profiler
is an open-source module in Python that shows line-by-line code analysis and the amount of memory consumed at each line of code.
It should be installed first using the following command:
#Python 3.x
pip install -U memory_profiler
To analyze the code put the function decorator @profile
above the function, we need to analyze.
# Python 3.x
from memory_profiler import profile
@profile
def myfunc():
var_a = [1] * (10 ** 6)
var_b = [2] * (2 * 10 ** 7)
del var_b
del var_a
myfunc()
We will pass the command line argument -m memory_profiler
to the Python interpreter when we run the code to display the memory consumption.
# Python 3.x
python -m memory_profiler filename.py
Output:
As we can see, the line number
, memory usage
, and memory
increment at each line of code.
Monitor Memory Consumption Using the guppy
Module in Python
The guppy
module is simple and easy for memory profiling. We will write the code for guppy at some point in our code to monitor the memory consumption.
It should be installed first using the following command:
#Python 3.x
pip install guppy3
To access Python’s heapy
object, we will call hpy()
. We will mark a reference point using setref()
to monitor the memory consumption.
To print the table, we will call the heap()
function with the heap object that we have created, and to get the total number of bytes consumed, we access the size attribute of heap status.
# Python 3.x
from guppy import hpy
heap = hpy()
heap.setref()
heap_status1 = heap.heap()
print("Heap Size before creating objects:", heap_status1.size, " bytes\n")
print(heap_status1)
a = []
for i in range(1000):
a.append(i)
heap_status2 = heap.heap()
print("Heap Size after creating objects : ", heap_status2.size, " bytes\n")
print(heap_status2)
Output:
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn