How to Fix Memory Error in Python
- Python Memory Error Due to Low RAM
- Python Memory Error Due to Wrong Python Version
- Python Memory Error Due to Unnecessary Object Creation
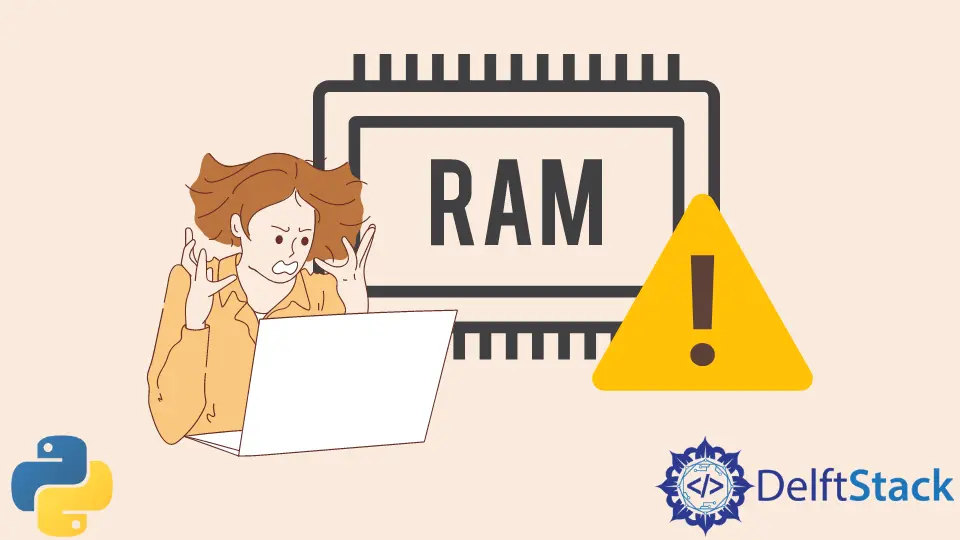
This tutorial will explain the memory error in Python, why it occurs, and how to prevent it.
Python Memory Error Due to Low RAM
The memory error occurs when the program runs out of memory, which means that either the PC’s memory is very low or the program is using unnecessary memory. If the reason behind the memory error is the low PC RAM, we can not do much about it other than upgrading the PC’s RAM, so that the program can run properly.
The user can also try to implement the programming practices explained below to prevent memory error.
Python Memory Error Due to Wrong Python Version
In case we have enough memory available but the program still gets out of memory, then the reason can be that Python cannot access the complete memory of the PC.
And the reason Python can not access complete memory can be that the user is using a 32-bit version of Python on a 64-bit machine. The user just needs to install the correct version of Python on the machine to resolve the error.
Python Memory Error Due to Unnecessary Object Creation
If the PC has enough RAM and has the correct version of Python, then the problem is probably within the code.
Like program is creating too many objects or performing unnecessary duplication. This can happen when we try to create all the objects in the program at once, or the program is creating new objects instead of reusing or deleting old ones. For example, the code creates a new object whenever the condition is true and does not delete the old objects.
Another reason for the memory error can be that the program is trying to load a huge file or dataset at once instead of loading it chunk by chunk. And in some cases, code keeps duplicating the same data instead of using its reference, which can also result in an out-of-memory error.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python