Max Int in Python
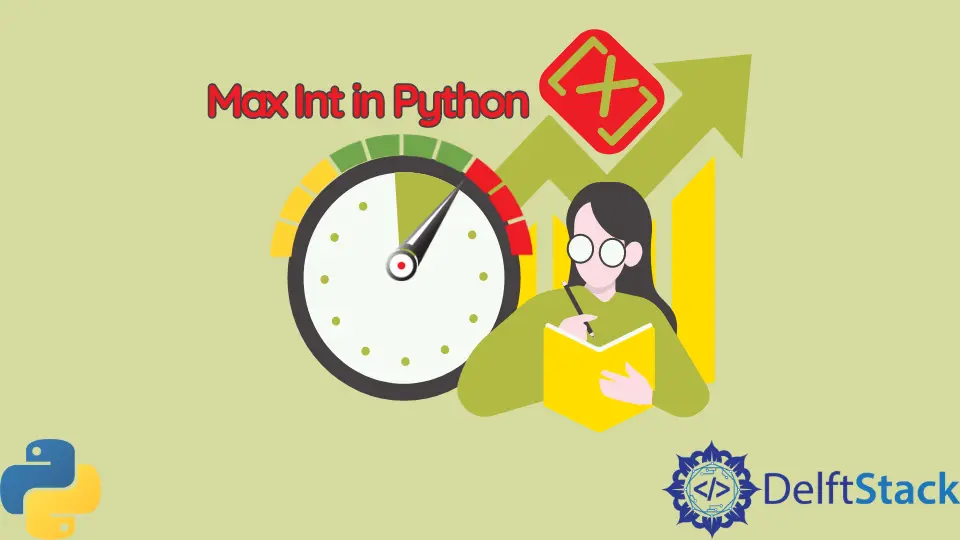
This tutorial will discuss the maximum integer value in different versions of Python and how we can get it.
In Python 2, an integer and a long integer are different data types. An integer has a limit of 231 - 1, and if the value exceeds the limit, the data type of the variable will automatically switch to long, and no exception will be raised. Once the variable data type is switched to long data type, it could be as large as the machine can store means there is no explicit limit for long in Python 2.
In Python 3, we do not have the separated integer and long datatypes, which means that the variable datatype will not be switched after the limit of 231 - 1 is exceeded. In short, the integer in Python 3 works in the same way as long does in Python 2 and can have a valu as large as the machine can store.
Get Maximum Integer Value in Python Using the sys
Module
As we have discussed above, there is no limit or maximum value of an integer in Python 3, but there is a limit for integer in Python 2, after which the data type of the variable switches to a long data type.
We can get the maximum integer value in Python 2 by using the sys.maxint
, equal to 231 - 1. The below example code demonstrates how to use the sys.maxint
to get the maximum integer value in Python 2:
import sys
print(sys.maxint)
Output:
9223372036854775807
In Python 3, the sys.maxint
does not exist as there is no limit or maximum value for an integer data type. But we can use sys.maxsize
to get the maximum value of the Py_ssize_t
type in Python 2 and 3. It is also the maximum size lists, strings, dictionaries, and similar container types can have.
The below example code demonstrates how to get the maximum value for container types in Python.
import sys
print(sys.maxsize)
In case we need to get the maximum or minimum value of the integer to use it in the condition check, we can use the float('inf')
and float('-inf')
to get the positive and negative infinity in Python.
Example code:
print(float("inf"))
print(float("-inf"))
Output:
inf
-inf