How to Fix Python Matplotlib Inline Invalid Syntax
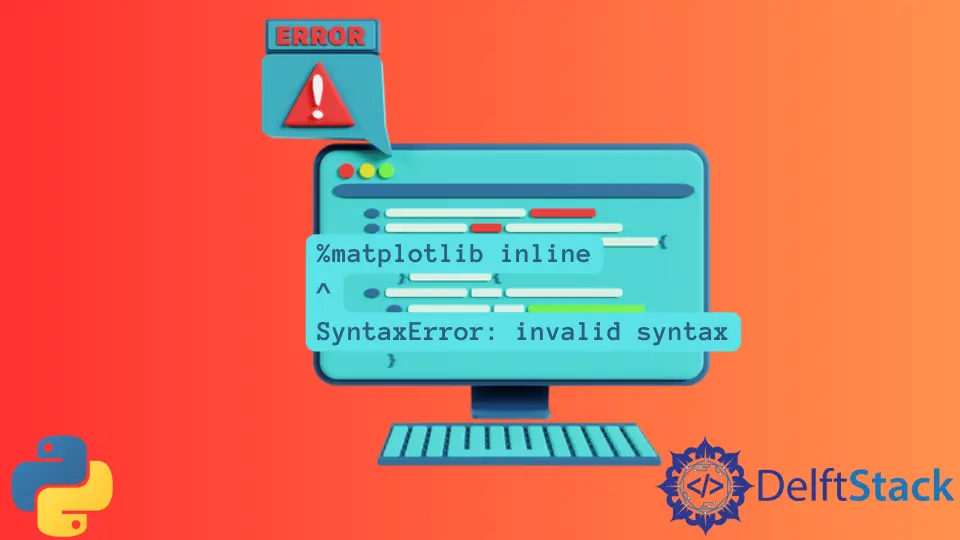
In this article, we’ll discuss why we get an error that is inline invalid syntax in Python and how to fix it.
Fix Matplotlib Inline Invalid Syntax Error in Python
One common mistake that beginners usually make is using the %matplotlib inline
magic function in VS code editor or another editor and getting a syntax error because this command only works in Jupyter Notebook. We can not use the %matplotlib inline
inside the other editor.
There is a way we can open a Jupyter Notebook inside the VS code. Open the extensions view by clicking on the extension’s icon and search for Python.
Install the Python extension from Microsoft; when the installation is complete, you may be prompted to select a Python interpreter, and you will be needed to select it.
Now we can create our first notebook, click on View
in the menu, select Command Palette, type a notebook name with its extension, and select create a new blank notebook
. We can also open the existing Jupyter Notebook in the VS code by right-clicking on the notebook and opening it with VS code.
Code:
%matplotlib inline
Output:
%matplotlib inline
^
SyntaxError: invalid syntax
When we open the Jupyter Notebook in VS code and run this command, it will execute successfully.
Using a Jupyter Notebook to create a plot with Matplotlib, you do not need to use the show()
method. One reason to use the inline
function is to display the plot below the code.
Another alternative is instead of using the %matplotlib inline
, we can use the show()
method inside the py
file.
Do not become frustrated if you are having trouble identifying a syntax error. Most people consider Python syntax mistakes to be the least specific form of error, and they are not always obvious.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python