How to Fix Python Math Domain Errors in math.log Function
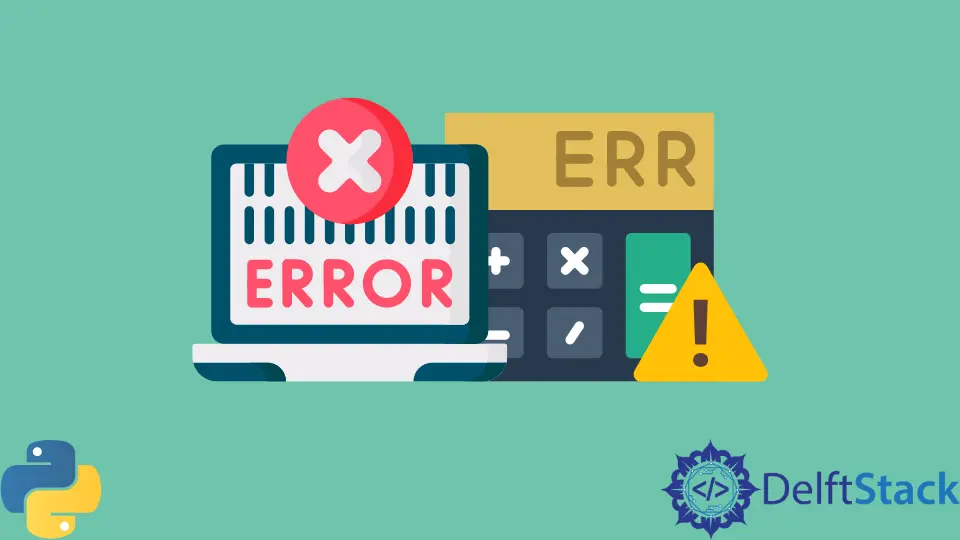
The ValueError
is raised in Python when the function receives a valid argument, but it is an inappropriate value. For instance, if you provide a negative number to the sqrt()
function, it returns ValueError
.
Similarly, if the specified value is 0 or a negative number in the math.log()
function, it throws ValueError
. This tutorial will teach you to solve ValueError: math domain error
in Python.
Fix the ValueError: math domain error
in math.log
Function Using Python
The math.log()
function computes the natural logarithm of a number or the logarithm of a number to the base. The syntax of math.log()
is as follows:
math.log(x, base)
Here, x
is a required value for which the logarithm will be calculated, while base
is an optional parameter. After importing the math
module, we can use the math.log()
function.
When you pass a negative number of a zero value to the math.log()
function, it returns ValueError
. It is because the logarithms of such numbers are mathematically undefined.
import math
print(math.log(-2))
Output:
Traceback (most recent call last):
File "c:\Users\rhntm\myscript.py", line 2, in <module>
print(math.log(-2))
ValueError: math domain error
We can resolve this error by passing a valid input value to the math.log()
function.
import math
print(math.log(2))
Output:
0.6931471805599453
You can use the decimal
module for passing a value close to zero to the math.log()
function. The ln
method of a Decimal
class calculates the natural logarithm of a decimal value.
import math
import decimal
num = decimal.Decimal("1E-1024")
print(math.log(num))
Output:
Traceback (most recent call last):
File "c:\Users\rhntm\myscript.py", line 5, in <module>
print(math.log(num))
ValueError: math domain error
Now, let’s use the Decimal
ln()
method.
from decimal import Decimal
num = Decimal("1E-1024")
print(num.ln())
Output:
-2357.847135225902780434423250
Now you know the reasons for ValueError: math domain error
in Python’s math.log()
function. The ValueError
occurs when you use an invalid input value in the function, which can be solved by passing a valid value to the function.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python