How to Convert a List to Lowercase in Python
-
Use the
str.lower()
Function and afor
Loop to Convert a List of Strings to Lowercase in Python -
Use the
map()
Function to Convert a List of Strings to Lowercase in Python - Use the Method of List Comprehension to Convert a List of Strings to Lowercase in Python
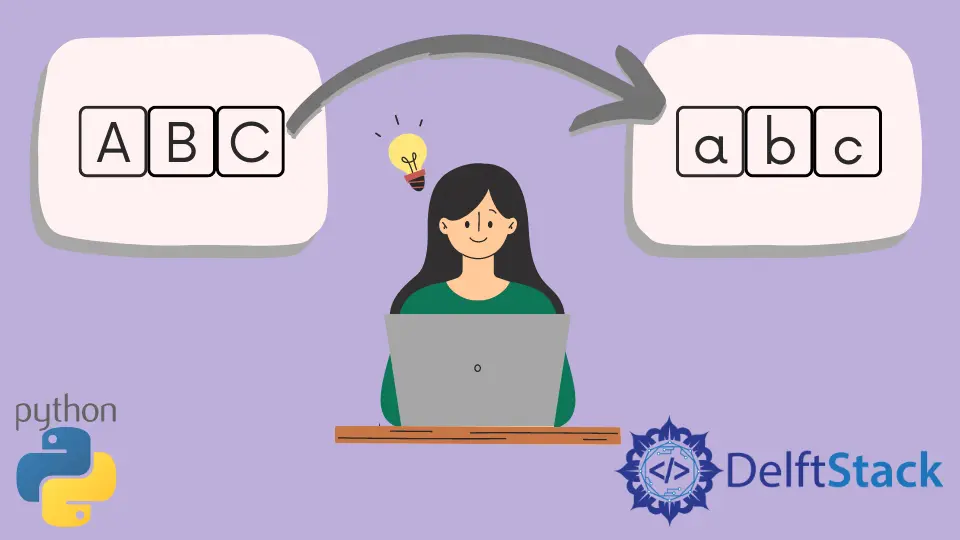
Lists can be utilized to stock multiple items in a single variable. In Python, we can create a list of strings in which the different elements contained in the list are enclosed within single or double quotes.
This tutorial demonstrates how to convert a list of strings to lowercase in Python.
Use the str.lower()
Function and a for
Loop to Convert a List of Strings to Lowercase in Python
The str.lower()
method is utilized to simply convert all uppercase characters in a given string into lowercase characters and provide the result. Similarly, the str.upper()
method is used to reverse this process.
Along with the str.lower()
function, the for
loop is also used to iterate all the elements in the given list of strings.
The following code uses the str.lower()
function and the for
loop to convert a list of strings to lowercase.
s = ["hEllO", "iNteRneT", "pEopLe"]
for i in range(len(s)):
s[i] = s[i].lower()
print(s)
Output:
["hello", "internet", "people"]
Use the map()
Function to Convert a List of Strings to Lowercase in Python
Python provides a map()
function, which can be utilized to apply a particular process among the given elements in any specified iterable; this function returns an iterator itself as the output.
A lambda function can be defined as a compact anonymous function that takes any amount of arguments and consists of only one expression. The lambda function will also be used along with the map
function in this method.
The following code uses the map()
function and the lambda function to convert a list of strings to lowercase in Python.
s = ["hEllO", "iNteRneT", "pEopLe"]
a = map(lambda x: x.lower(), s)
b = list(a)
print(b)
Output:
["hello", "internet", "people"]
Use the Method of List Comprehension to Convert a List of Strings to Lowercase in Python
List comprehension is a much shorter way to create lists to be formed based on the given values of an already existing list. This method basically creates a new list in which all the items are lowercased.
The following code uses list comprehension to convert a list of strings to lowercase.
s = ["hEllO", "iNteRneT", "pEopLe"]
a = [x.lower() for x in s]
print(a)
Output:
['hello', 'internet', 'people']
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python