How to Loop Through a List in Python
-
Loop Through a Python List Using the
for
Loop - Loop Through a Python List Using the List Comprehension Method
-
Loop Through a Python List From and Till Specific Indexes Using the
range()
Function
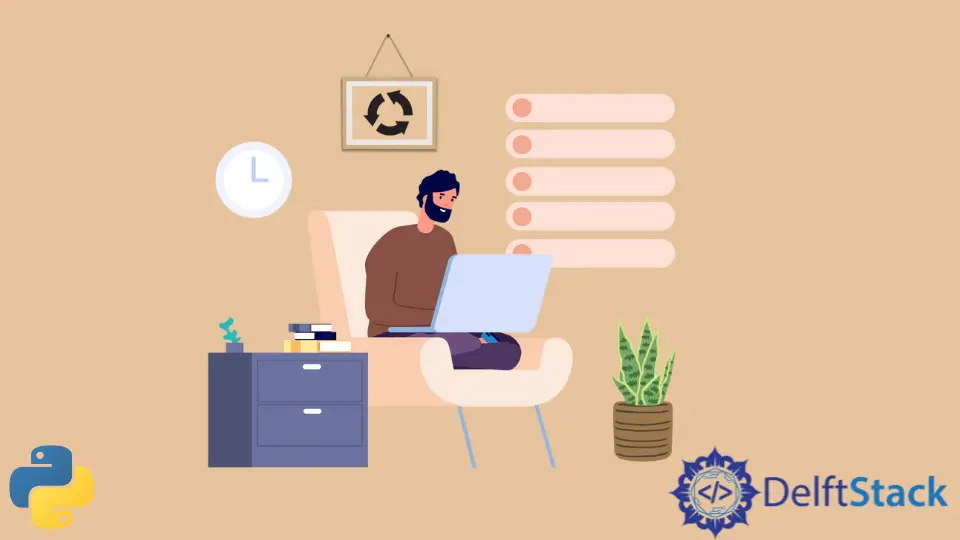
This tutorial will explain various methods to loop through the list in Python. Looping through a list or other iterable object is a very commonly used functionality in programming to save and access data in it or perform some actions on the data saved in the list.
This tutorial will look into different ways to iterate through a list from start to end and start iteration from a specific index.
Loop Through a Python List Using the for
Loop
One simple way to loop through a list or any iterable object in Python is by using the for
loop. The below example code demonstrates how to use the for
loop to iterate through a list in Python.
mylist = [1, 4, 7, 3, 21]
for x in mylist:
print(x)
Output:
1
4
7
3
21
Loop Through a Python List Using the List Comprehension Method
List comprehension is a syntactic way to create a new list from an existing list. This method is useful if we want to perform some operation on the elements of the list while looping through them. Like replacing some specific elements, applying some mathematical functions like divide, multiply, log or exponential, etc., or removing some specific elements from the list.
The below example code shows how to loop through a list and remove odd numbers using the list comprehension involving a one line for
loop in Python.
mylist = [1, 4, 7, 8, 20]
newlist = [x for x in mylist if x % 2 == 0]
print(newlist)
Output:
[4, 8, 20]
Loop Through a Python List From and Till Specific Indexes Using the range()
Function
In case we want to loop through the list from and to some specific index, we can do so using the range()
function.
The range(start, stop, step)
function returns a sequence that starts from the start
value, ends at the stop
value, and takes the step equal to the step
argument whose default value 1
.
The below example code demonstrates how to use the range()
function to loop through a specific range of indexes of the list in Python.
mylist = ["a", "b", "c", "d", "e", "f", "g"]
for x in range(2, len(mylist) - 1):
print(mylist[x])
Output:
c
d
e
f
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python