Logical AND Operator in Python
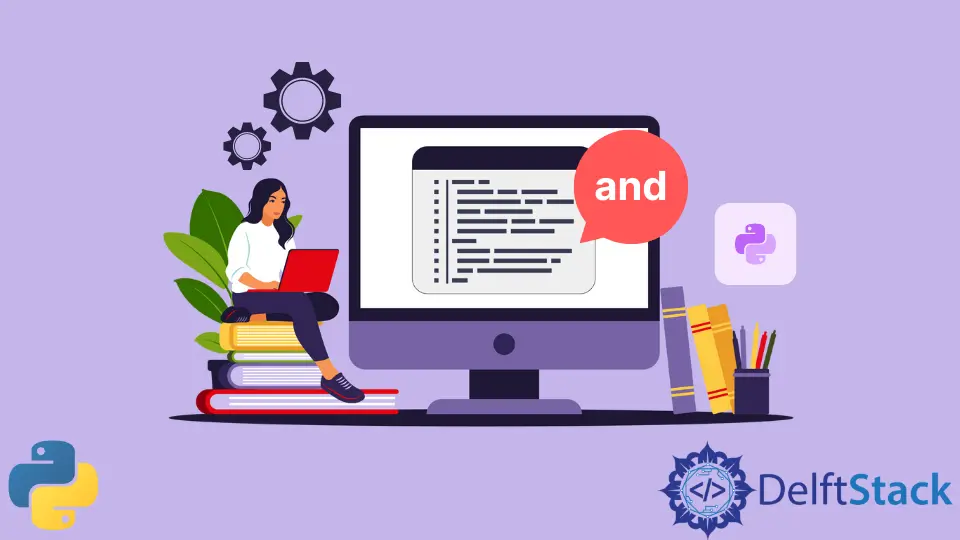
This tutorial will explain the syntax and use of the logical AND operator in Python. The logical AND operator returns True
if the value of both operands is True
, and returns False
if any value of the two operands is False
. The logical AND operator is used in cases where we only want to perform an action or a task if all the conditions or operands are True
.
In most programming languages, i.e. C, C++, Java, and C#, etc. &&
is used as a logical AND operator. Unlike other programming languages, the and
keyword is used as the logical AND operator in Python.
Examples of Logical and Operator and
in Python
Now let’s look into the use with example codes of the logical and operator and
in Python.
Suppose we have a program that performs actions based on the two variables a
and b
; we check values of a
and b
using the and
keyword, as shown in the below example code.
pythonCopya = 12
b = 2
if a > 0 and b > 0:
print("a and b are greater than 0")
Output:
textCopya and b are greater than 0
Another use of the and
keyword can be where we want to check the functions’ outputs and then perform an action or task based on boolean values returned by the values.
The below example code demonstrates the use of the logical AND operator and
in Python to check the boolean values returned by the functions.
pythonCopyfunc1 = True
func2 = False
if func1 and func2:
print("Both function executed successfully")
else:
print("Task failed")
Output:
textCopyTask failed
We can also check the values of more than two operands, i.e. if all the conditions are True
using multiple logical AND operators and
in Python as shown in the example code below:
pythonCopycond1 = True
cond2 = True
cond3 = False
cond4 = True
if cond1 and cond2 and cond3 and cond4:
print("All conditions are true!")
else:
print("All conditions are not satisfied")
Output:
textCopyAll conditions are not satisfied