How to Propagate Logging in Python
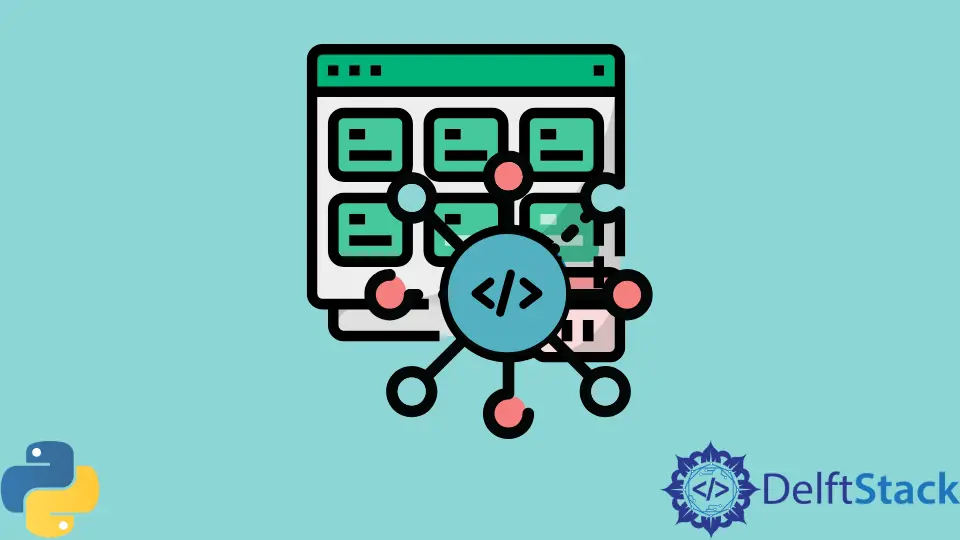
This tutorial demonstrates the use of LevelFilter
to show how to log messages of a particular logger name of a particular level and above (for instance, INFO
and above) to a specific log handler.
Python Logging Propagate
We have already written an article talking about the logging
module, its importance, various logging levels, and demonstrate the local and global use of logging
module. Here, we will learn how we can propagate specific messages of the level below the current logger level.
What does that mean? It means we want to log messages of a particular logger name, of a specific level and higher (let’s say INFO
and up) to a particular log handler (let’s assume a stream handler or a file handler).
Example Code:
import logging
class LevelFilter(logging.Filter):
def __init__(self, level):
self.level = level
def filter(self, record):
return record.levelno >= self.level
def perform_logging(turn):
logger = logging.getLogger("extra")
logger.info("some information turn %d" % turn)
logger.debug("this is the debug fudge turn %d" % turn)
rootLogger = logging.getLogger()
handler = logging.StreamHandler()
rootFormatter = logging.Formatter("root - %(levelname)s: %(msg)s")
handler.setFormatter(rootFormatter)
rootLogger.addHandler(handler)
rootLogger.setLevel(logging.DEBUG)
perform_logging(1)
extraLogger = logging.getLogger("extra")
extraHandler = logging.StreamHandler()
extraFormatter = logging.Formatter("extra - %(levelname)s: %(msg)s")
extraHandler.setFormatter(extraFormatter)
extraLogger.addHandler(extraHandler)
extraHandler.addFilter(LevelFilter(logging.INFO))
extraLogger.setLevel(logging.DEBUG)
perform_logging(2)
OUTPUT:
root - INFO: some information turn 1
root - DEBUG: this is the debug fudge turn 1
extra - INFO: some information turn 2
root - INFO: some information turn 2
root - DEBUG: this is the debug fudge turn 2
In this example, we create two loggers: a root
logger and a named logger (which is extra
here).
We attach the logging.StreamHandler
for a root
logger and set the log level to logging.DEBUG
. Afterward, we attach a handler to a named logger and set its logging level to logging.INFO
.
Now the point is how we propagate messages of a specific level below the current level. To do that, we use LevelFilter
to add a filter to every handler that allows the particular level only.