How to Perform List Subtraction in Python
-
Convert List to
set
to Perform List Subtraction in Python - Use List Comprehension to Get List Difference in Python
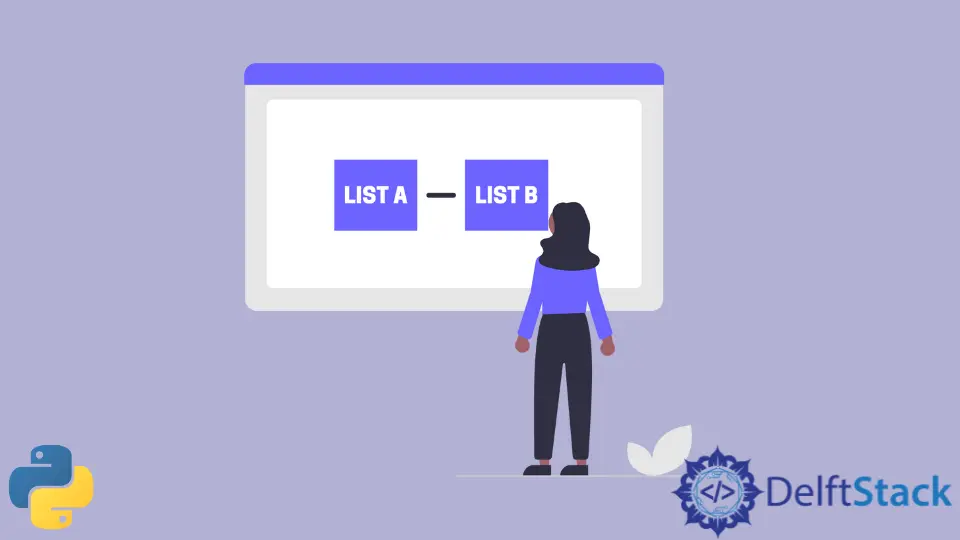
This tutorial demonstrates how to perform the list subtraction, or in other words, list minus list in Python.
As defined by the set theory in mathematics, the difference of two sets refers to the elements from one set that do not exist in the other set.
For example, if we declare these two lists:
list1 = [1, 2, 4]
list2 = [2, 3]
The difference of list1 - list2
would be [1, 4]
, while list2 - list1
would be [3]
.
Convert List to set
to Perform List Subtraction in Python
Set theory operations are supported in Python. However, only the set
data type support these operations. Therefore, to use the set
operation, lists have to be converted into sets. This is possible by wrapping a list around the function set()
.
listA = [1, 2, 4, 7, 9, 11, 11, 14, 14]
listB = [2, 3, 7, 8, 11, 13, 13, 16]
setA = set(listA)
setB = set(listB)
print("A - B = ", setA - setB)
Output:
A - B = {1, 4, 9, 14}
The result outputs the difference between the two sets and removes the duplicate values.
We can use the function list()
to convert the result from a set
to a list.
listA = [1, 2, 4, 7, 9, 11, 11, 14, 14]
listB = [2, 3, 7, 8, 11, 13, 13, 16]
setA = set(listA)
setB = set(listB)
list_diff = list(setA - setB)
print("A - B: ", list_diff)
Output:
A - B: [1, 4, 9, 14]
Use List Comprehension to Get List Difference in Python
List comprehension can be used to check if an element exists only in the first list but does not exist in the second list. This solution makes it possible to perform the difference operation without converting the list to a set.
listA = [1, 2, 4, 7, 9, 11, 11, 14, 14]
listB = [2, 3, 7, 8, 11, 13, 13, 16]
listSub = [elem for elem in listA if elem not in listB]
print("A - B =", listSub)
Output:
A - B = [1, 4, 9, 14, 14]
This solution does not mess with the order of the list and removes duplicates.
However, the value 11
is repeated twice in listA
, and both iterations of 11
are removed from the result of A - B
since 11
exists in both sets. This behavior is as expected.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python