if...else in Python List Comprehension
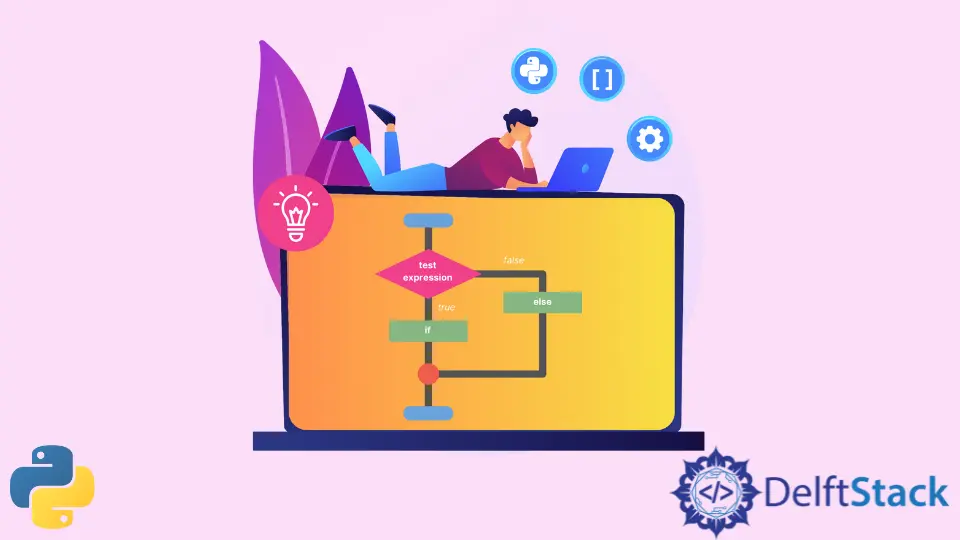
This tutorial will explain multiple ways to perform list comprehension with the if ... else
statement in Python. A list comprehension is a short and syntactic way to create a list based on an existing list. It is usually used to create a new filtered or changed list from a current list.
For example, we have a python list ['Ali','Mark', None, 'Sara', None, 'Rahul']
and we want to create a new list ['Ali','Mark', '', 'Sara', '', 'Rahul']
, we can do it by using list comprehension.
Python if ... else
List Comprehension
The below example code demonstrates how we can create a changed list from the existing list using list comprehension with the if ... else
statement:
my_list = ["Ali", "Mark", None, "Sara", None, "Rahul"]
new_list = [str(x.strip()) if x is not None else "" for x in my_list]
print(new_list)
Output:
['Ali', 'Mark', '', 'Sara', '', 'Rahul']
The general syntax of list comprehension in Python with if ... else
is:
[f(x) if condition else g(x) for x in list]
If condition
is true for the list element x
, f(x)
, any applicable function, is applied to the element; otherwise, g(x)
will be applied.
Example code:
my_list = ["Ali", "Mark", None, "Sara", None, "Rahul"]
new_list = [x.upper() if x is not None else "" for x in my_list]
print(new_list)
Output:
['ALI', 'MARK', '', 'SARA', '', 'RAHUL']
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python