if...else in Lambda Function Python
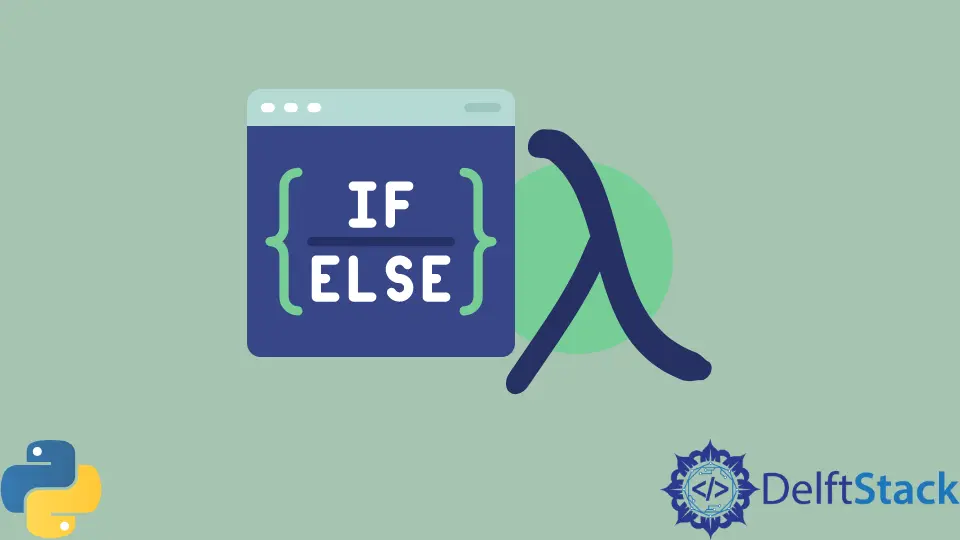
A lambda
function is used to implement some simple logic in Python and can be thought of as an anonymous function. It can have many arguments but can only have one expression, just like any other function defined using the def
keyword.
We can define lambda
functions in a single line of code and have proven to be very useful in Python.
In this tutorial, we will use the if-else
statement in lambda
functions in Python.
The if-else
statement is a conditional statement in Python used to execute both the True and False parts of the condition.
The code within the if
block will execute when the condition code is True, and the else
block when the condition is False.
We can incorporate the if-else
statements within a lambda
function in Python.
See the following example,
def test(a):
return True if (a > 10 and a < 20) else False
print(test(13))
print(test(1))
print(test(34))
Output:
True
False
False