How to Fix Keywords Cannot Be Expression Error in Python
- Understanding the Keywords Cannot Be Expression Error
- Common Causes of the Error
- Fixing the Error by Renaming Variables
- Fixing the Error by Checking Parentheses
- Fixing the Error by Reviewing Function Calls
- Conclusion
- FAQ
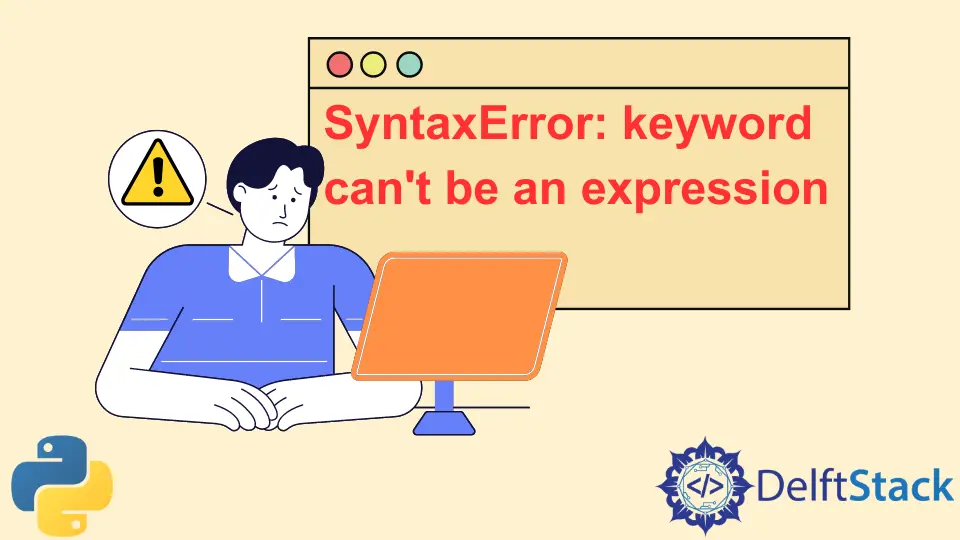
In the world of programming, encountering errors is an inevitable part of the journey. One such common issue is the “keywords cannot be expression” error in Python. This error typically arises when you mistakenly use a keyword in a context where an expression is expected. It can be frustrating, especially for beginners, as it halts your progress. However, fear not!
This tutorial is designed to guide you through understanding and fixing this error effectively. By the end, you’ll have a clearer grasp of how to avoid this pitfall and enhance your coding skills. Let’s dive into the details and explore practical solutions to resolve this error.
Understanding the Keywords Cannot Be Expression Error
Before we jump into solutions, let’s clarify what the “keywords cannot be expression” error means. In Python, keywords are reserved words that have special meanings and cannot be used as identifiers for variables, functions, or classes. When you attempt to use a keyword inappropriately, Python raises an error indicating that it expected an expression but found a keyword instead.
For example, if you try to use if
or else
as a variable name, Python will throw this error. Understanding this concept is crucial for avoiding such issues in your code. Now, let’s look at some practical examples and solutions to fix this error.
Common Causes of the Error
The error can occur in various scenarios, often due to simple mistakes. Here are some common causes:
- Using a keyword as a variable name: This is the most frequent cause. For instance, using
def
orclass
as variable names will trigger the error. - Misplacing parentheses: Sometimes, incorrect placement of parentheses can lead to confusion in the code structure, causing Python to misinterpret your intentions.
- Improper function calls: If you attempt to call a function using a keyword, it can lead to this error.
Recognizing these common pitfalls will help you avoid the “keywords cannot be expression” error in the future.
Fixing the Error by Renaming Variables
One of the simplest and most effective ways to fix the “keywords cannot be expression” error is by renaming your variables. If you find yourself using a keyword as a variable name, simply choose a different name that is not reserved. Here’s an example to illustrate this:
def = 5
print(def)
This code will raise an error because def
is a keyword in Python. To fix it, you can rename the variable:
my_var = 5
print(my_var)
Output:
5
By changing def
to my_var
, the error is resolved. This simple adjustment allows your code to run smoothly. Always remember to check your variable names against Python’s list of keywords to avoid this pitfall.
Fixing the Error by Checking Parentheses
Another common source of the “keywords cannot be expression” error is misplaced parentheses. When parentheses are not correctly placed, Python might misinterpret your code structure, leading to confusion. For instance:
if (x > 10
print("X is greater than 10")
In the above code, the parentheses are incorrectly placed. Here’s the corrected version:
if (x > 10):
print("X is greater than 10")
Output:
X is greater than 10
By ensuring that your parentheses are correctly placed, you can avoid syntax errors. Always double-check your code for proper syntax, especially when using control structures like if
statements.
Fixing the Error by Reviewing Function Calls
Improper function calls can also lead to the “keywords cannot be expression” error. When calling a function, ensure that you are not using keywords as arguments or in the function name. For example:
def my_function():
return "Hello, World!"
my_function = my_function()
print(my_function)
In this snippet, we mistakenly overwrite the function name. Here’s how to fix it:
def my_function():
return "Hello, World!"
result = my_function()
print(result)
Output:
Hello, World!
By assigning the result of the function call to a different variable (result
), we avoid the error. This practice not only resolves the issue but also enhances code readability.
Conclusion
The “keywords cannot be expression” error in Python can be a stumbling block for many programmers, but with a little understanding and the right strategies, you can easily overcome it. By renaming variables, checking parentheses, and reviewing function calls, you can ensure that your code runs smoothly. Remember, the key to mastering Python is practice and learning from your mistakes. Keep coding, and you’ll find that these errors become increasingly rare as you grow in your programming journey.
FAQ
-
What are Python keywords?
Python keywords are reserved words that have special meaning in the language. They cannot be used as identifiers for variables, functions, or classes. -
How can I find a list of Python keywords?
You can find the list of Python keywords by using thekeyword
module. Simply import it and use thekeyword.kwlist
command. -
Can I use keywords as part of variable names?
No, you cannot use keywords as variable names, but you can use them as part of a longer variable name as long as the full name is not a keyword. -
What should I do if I encounter this error?
Check your variable names, ensure proper parentheses placement, and review your function calls for any misuse of keywords. -
Is this error common among beginners?
Yes, this error is quite common among beginners who are still getting familiar with Python’s syntax and reserved keywords.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python